# Data Structure Typed






[//]: # ()
Data Structures of Javascript & TypeScript.
Do you envy C++ with [STL](), Python with [collections](), and Java with [java.util]() ? Well, no need to envy anymore! JavaScript and TypeScript now have [data-structure-typed]().
Now you can use this library in Node.js and browser environments in CommonJS(require export.modules = ), ESModule(import export), Typescript(import export), UMD(var Queue = dataStructureTyped.Queue)
[//]: # ()
[//]: # ()
[//]: # ()
[//]: # ()
## Installation and Usage
### npm
```bash
npm i data-structure-typed
```
### yarn
```bash
yarn add data-structure-typed
```
```js
import {
BinaryTree, Graph, Queue, Stack, PriorityQueue, BST, Trie, DoublyLinkedList,
AVLTree, MinHeap, SinglyLinkedList, DirectedGraph, TreeMultimap,
DirectedVertex, AVLTreeNode
} from 'data-structure-typed';
```
### CDN
Copy the line below into the head tag in an HTML document.
#### development
```html
```
#### production
```html
```
Copy the code below into the script tag of your HTML, and you're good to go with your development work.
```js
const {Heap} = dataStructureTyped;
const {
BinaryTree, Graph, Queue, Stack, PriorityQueue, BST, Trie, DoublyLinkedList,
AVLTree, MinHeap, SinglyLinkedList, DirectedGraph, TreeMultimap,
DirectedVertex, AVLTreeNode
} = dataStructureTyped;
```
## Software Engineering Design Standards
Principle |
Description |
Practicality |
Follows ES6 and ESNext standards, offering unified and considerate optional parameters, and simplifies method names. |
Extensibility |
Adheres to OOP (Object-Oriented Programming) principles, allowing inheritance for all data structures. |
Modularization |
Includes data structure modularization and independent NPM packages. |
Efficiency |
All methods provide time and space complexity, comparable to native JS performance. |
Maintainability |
Follows open-source community development standards, complete documentation, continuous integration, and adheres to TDD (Test-Driven Development) patterns. |
Testability |
Automated and customized unit testing, performance testing, and integration testing. |
Portability |
Plans for porting to Java, Python, and C++, currently achieved to 80%. |
Reusability |
Fully decoupled, minimized side effects, and adheres to OOP. |
Security |
Carefully designed security for member variables and methods. Read-write separation. Data structure software does not need to consider other security aspects. |
Scalability |
Data structure software does not involve load issues. |
## Vivid Examples
### Binary Tree
[Try it out](https://vivid-algorithm.vercel.app/), or you can execute your own code using our [visual tool](https://github.com/zrwusa/vivid-algorithm)
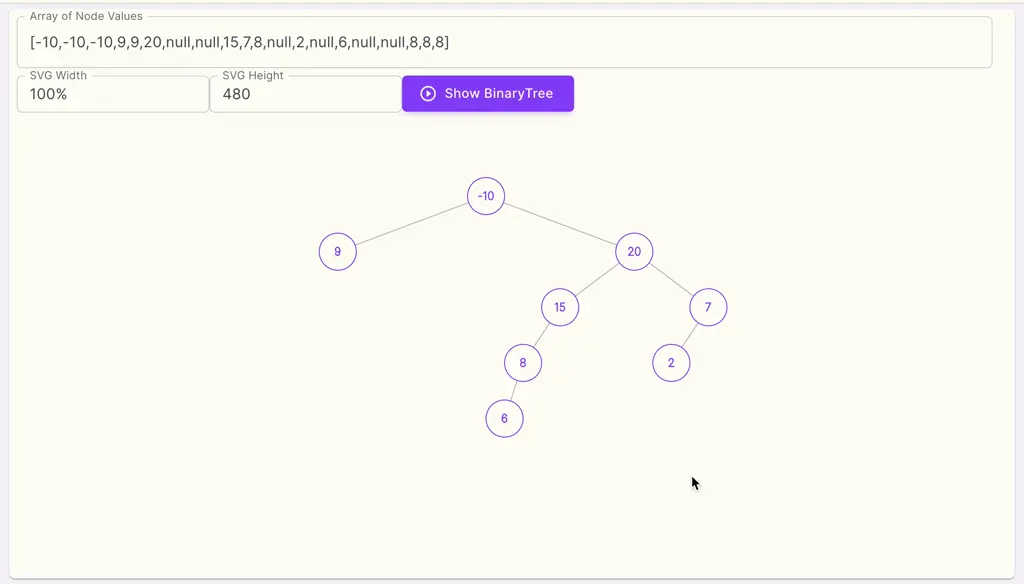
### Binary Tree DFS
[Try it out](https://vivid-algorithm.vercel.app/), or you can execute your own code using our [visual tool](https://github.com/zrwusa/vivid-algorithm)
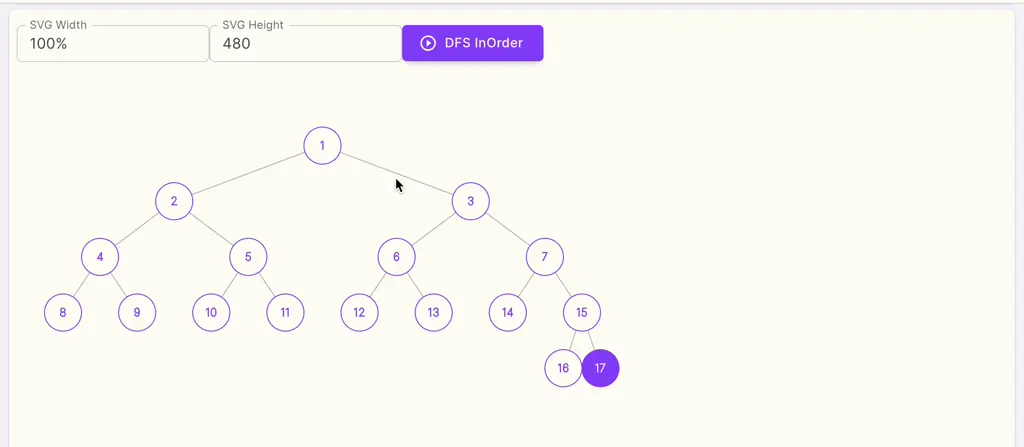
### AVL Tree
[Try it out](https://vivid-algorithm.vercel.app/), or you can execute your own code using our [visual tool](https://github.com/zrwusa/vivid-algorithm)
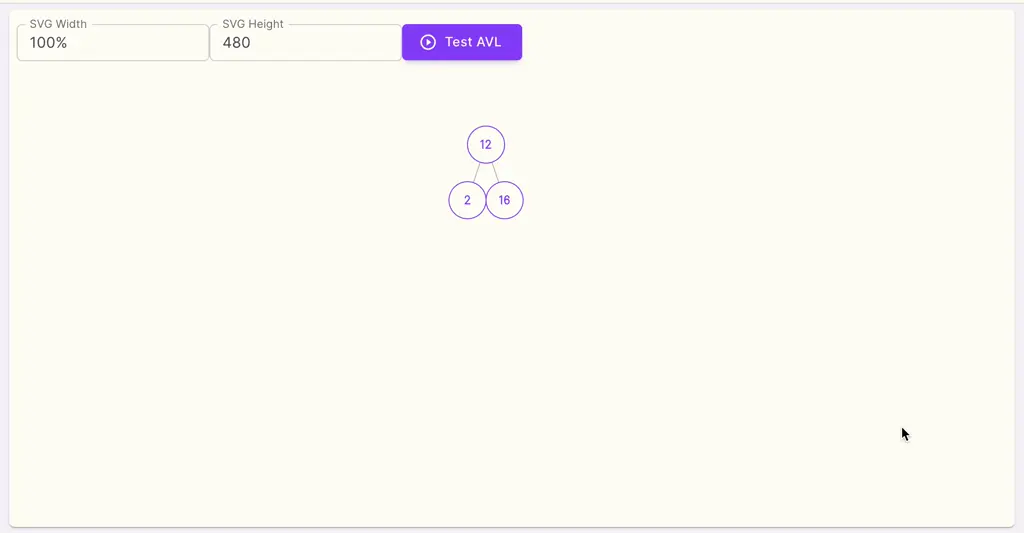
### Tree Multi Map
[Try it out](https://vivid-algorithm.vercel.app/), or you can execute your own code using our [visual tool](https://github.com/zrwusa/vivid-algorithm)
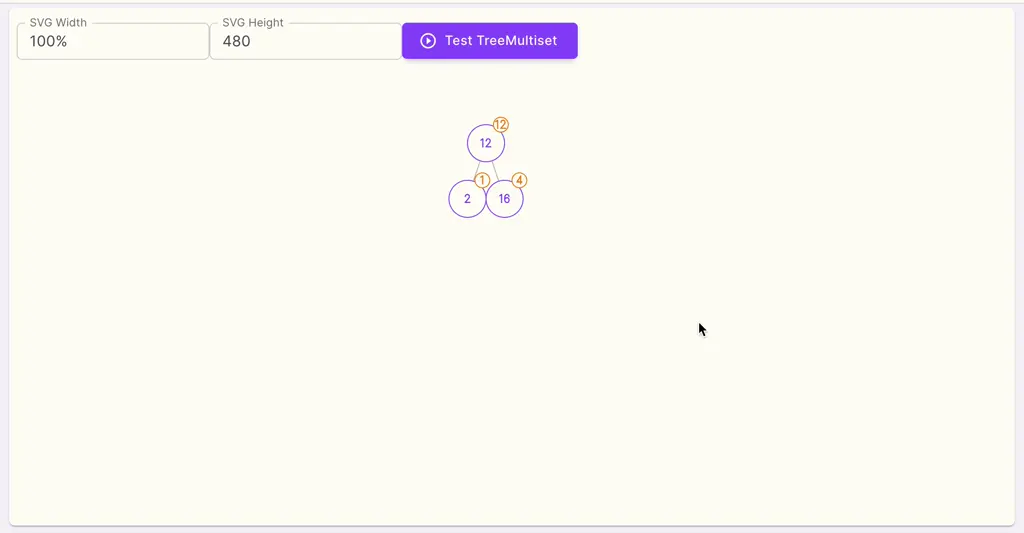
### Matrix
[Try it out](https://vivid-algorithm.vercel.app/algorithm/graph/), or you can execute your own code using our [visual tool](https://github.com/zrwusa/vivid-algorithm)
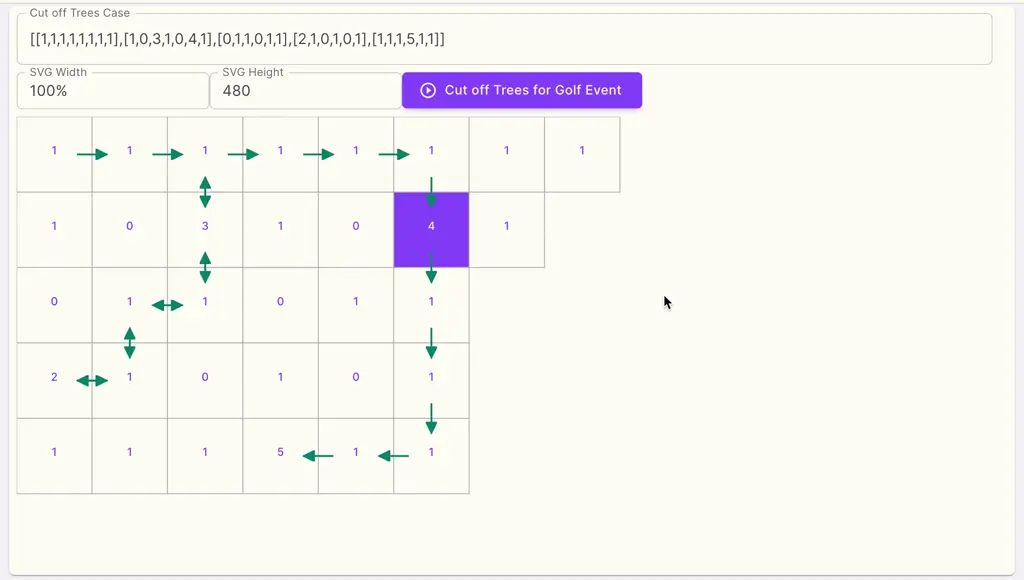
### Directed Graph
[Try it out](https://vivid-algorithm.vercel.app/algorithm/graph/), or you can execute your own code using our [visual tool](https://github.com/zrwusa/vivid-algorithm)
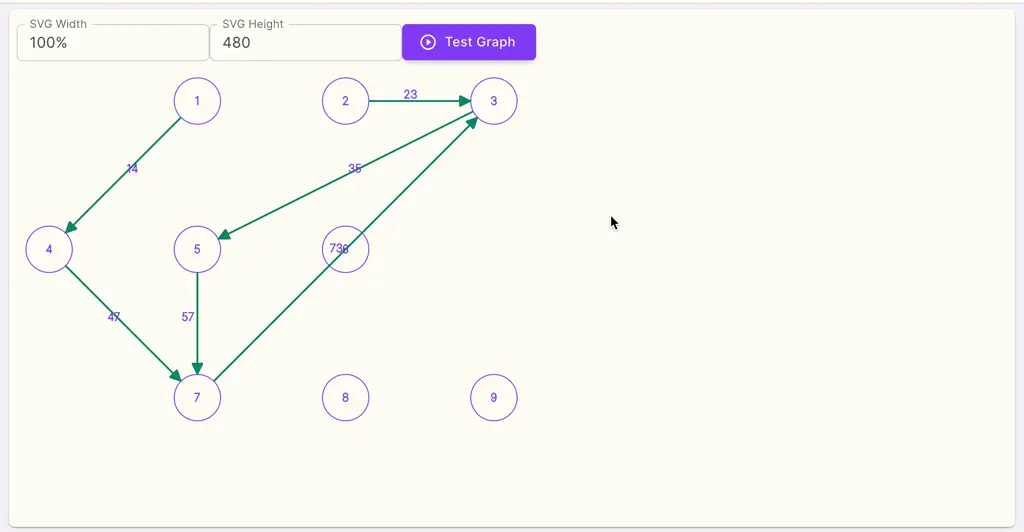
### Map Graph
[Try it out](https://vivid-algorithm.vercel.app/algorithm/graph/), or you can execute your own code using our [visual tool](https://github.com/zrwusa/vivid-algorithm)
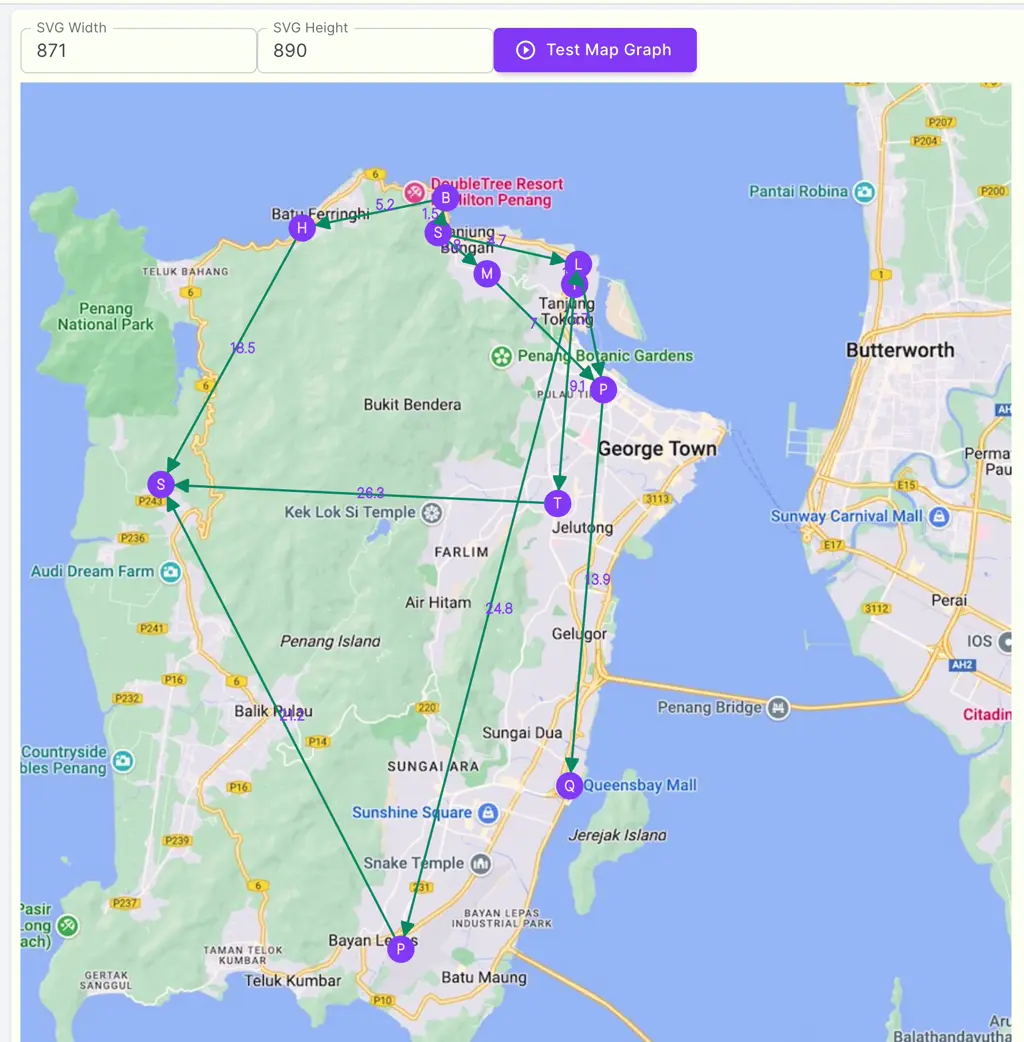
## Code Snippets
### Binary Search Tree (BST) snippet
#### TS
```ts
import {BST, BSTNode} from 'data-structure-typed';
const bst = new BST();
bst.add(11);
bst.add(3);
bst.addMany([15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5]);
bst.size === 16; // true
bst.has(6); // true
const node6 = bst.getNode(6); // BSTNode
bst.getHeight(6) === 2; // true
bst.getHeight() === 5; // true
bst.getDepth(6) === 3; // true
bst.getLeftMost()?.key === 1; // true
bst.delete(6);
bst.get(6); // undefined
bst.isAVLBalanced(); // true
bst.bfs()[0] === 11; // true
const objBST = new BST<{height: number, age: number}>();
objBST.add(11, { "name": "Pablo", "age": 15 });
objBST.add(3, { "name": "Kirk", "age": 1 });
objBST.addMany([15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5], [
{ "name": "Alice", "age": 15 },
{ "name": "Bob", "age": 1 },
{ "name": "Charlie", "age": 8 },
{ "name": "David", "age": 13 },
{ "name": "Emma", "age": 16 },
{ "name": "Frank", "age": 2 },
{ "name": "Grace", "age": 6 },
{ "name": "Hannah", "age": 9 },
{ "name": "Isaac", "age": 12 },
{ "name": "Jack", "age": 14 },
{ "name": "Katie", "age": 4 },
{ "name": "Liam", "age": 7 },
{ "name": "Mia", "age": 10 },
{ "name": "Noah", "age": 5 }
]
);
objBST.delete(11);
```
#### JS
```js
const {BST, BSTNode} = require('data-structure-typed');
const bst = new BST();
bst.add(11);
bst.add(3);
bst.addMany([15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5]);
bst.size === 16; // true
bst.has(6); // true
const node6 = bst.getNode(6);
bst.getHeight(6) === 2; // true
bst.getHeight() === 5; // true
bst.getDepth(6) === 3; // true
const leftMost = bst.getLeftMost();
leftMost?.key === 1; // true
bst.delete(6);
bst.get(6); // undefined
bst.isAVLBalanced(); // true or false
const bfsIDs = bst.bfs();
bfsIDs[0] === 11; // true
```
### AVLTree snippet
#### TS
```ts
import {AVLTree} from 'data-structure-typed';
const avlTree = new AVLTree();
avlTree.addMany([11, 3, 15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5])
avlTree.isAVLBalanced(); // true
avlTree.delete(10);
avlTree.isAVLBalanced(); // true
```
#### JS
```js
const {AVLTree} = require('data-structure-typed');
const avlTree = new AVLTree();
avlTree.addMany([11, 3, 15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5])
avlTree.isAVLBalanced(); // true
avlTree.delete(10);
avlTree.isAVLBalanced(); // true
```
### Directed Graph simple snippet
#### TS or JS
```ts
import {DirectedGraph} from 'data-structure-typed';
const graph = new DirectedGraph();
graph.addVertex('A');
graph.addVertex('B');
graph.hasVertex('A'); // true
graph.hasVertex('B'); // true
graph.hasVertex('C'); // false
graph.addEdge('A', 'B');
graph.hasEdge('A', 'B'); // true
graph.hasEdge('B', 'A'); // false
graph.deleteEdgeSrcToDest('A', 'B');
graph.hasEdge('A', 'B'); // false
graph.addVertex('C');
graph.addEdge('A', 'B');
graph.addEdge('B', 'C');
const topologicalOrderKeys = graph.topologicalSort(); // ['A', 'B', 'C']
```
### Undirected Graph snippet
#### TS or JS
```ts
import {UndirectedGraph} from 'data-structure-typed';
const graph = new UndirectedGraph();
graph.addVertex('A');
graph.addVertex('B');
graph.addVertex('C');
graph.addVertex('D');
graph.deleteVertex('C');
graph.addEdge('A', 'B');
graph.addEdge('B', 'D');
const dijkstraResult = graph.dijkstra('A');
Array.from(dijkstraResult?.seen ?? []).map(vertex => vertex.key) // ['A', 'B', 'D']
```
## Built-in classic algorithms
Algorithm |
Function Description |
Iteration Type |
Binary Tree DFS |
Traverse a binary tree in a depth-first manner, starting from the root node, first visiting the left subtree,
and then the right subtree, using recursion.
|
Recursion + Iteration |
Binary Tree BFS |
Traverse a binary tree in a breadth-first manner, starting from the root node, visiting nodes level by level
from left to right.
|
Iteration |
Graph DFS |
Traverse a graph in a depth-first manner, starting from a given node, exploring along one path as deeply as
possible, and backtracking to explore other paths. Used for finding connected components, paths, etc.
|
Recursion + Iteration |
Binary Tree Morris |
Morris traversal is an in-order traversal algorithm for binary trees with O(1) space complexity. It allows tree
traversal without additional stack or recursion.
|
Iteration |
Graph BFS |
Traverse a graph in a breadth-first manner, starting from a given node, first visiting nodes directly connected
to the starting node, and then expanding level by level. Used for finding shortest paths, etc.
|
Recursion + Iteration |
Graph Tarjan's Algorithm |
Find strongly connected components in a graph, typically implemented using depth-first search. |
Recursion |
Graph Bellman-Ford Algorithm |
Finding the shortest paths from a single source, can handle negative weight edges |
Iteration |
Graph Dijkstra's Algorithm |
Finding the shortest paths from a single source, cannot handle negative weight edges |
Iteration |
Graph Floyd-Warshall Algorithm |
Finding the shortest paths between all pairs of nodes |
Iteration |
Graph getCycles |
Find all cycles in a graph or detect the presence of cycles. |
Recursion |
Graph getCutVertexes |
Find cut vertices in a graph, which are nodes that, when removed, increase the number of connected components in
the graph.
|
Recursion |
Graph getSCCs |
Find strongly connected components in a graph, which are subgraphs where any two nodes can reach each other.
|
Recursion |
Graph getBridges |
Find bridges in a graph, which are edges that, when removed, increase the number of connected components in the
graph.
|
Recursion |
Graph topologicalSort |
Perform topological sorting on a directed acyclic graph (DAG) to find a linear order of nodes such that all
directed edges go from earlier nodes to later nodes.
|
Recursion |
## API docs & Examples
[API Docs](https://data-structure-typed-docs.vercel.app)
[Live Examples](https://vivid-algorithm.vercel.app)
Examples Repository
## Data Structures
## Standard library data structure comparison
Data Structure Typed |
C++ STL |
java.util |
Python collections |
DoublyLinkedList<E> |
list<T> |
LinkedList<E> |
deque |
SinglyLinkedList<E> |
- |
- |
- |
Array<E> |
vector<T> |
ArrayList<E> |
list |
Queue<E> |
queue<T> |
Queue<E> |
- |
Deque<E> |
deque<T> |
- |
- |
PriorityQueue<E> |
priority_queue<T> |
PriorityQueue<E> |
- |
Heap<E> |
priority_queue<T> |
PriorityQueue<E> |
heapq |
Stack<E> |
stack<T> |
Stack<E> |
- |
Set<E> |
set<T> |
HashSet<E> |
set |
Map<K, V> |
map<K, V> |
HashMap<K, V> |
dict |
- |
unordered_set<T> |
HashSet<E> |
- |
HashMap<K, V> |
unordered_map<K, V> |
HashMap<K, V> |
defaultdict |
Map<K, V> |
- |
- |
OrderedDict |
BinaryTree<K, V> |
- |
- |
- |
BST<K, V> |
- |
- |
- |
TreeMultimap<K, V> |
multimap<K, V> |
- |
- |
AVLTree<E> |
- |
TreeSet<E> |
- |
AVLTree<K, V> |
- |
TreeMap<K, V> |
- |
AVLTree<E> |
set |
TreeSet<E> |
- |
Trie |
- |
- |
- |
- |
multiset<T> |
- |
- |
DirectedGraph<V, E> |
- |
- |
- |
UndirectedGraph<V, E> |
- |
- |
- |
- |
unordered_multiset |
- |
Counter |
- |
- |
LinkedHashSet<E> |
- |
- |
- |
LinkedHashMap<K, V> |
- |
- |
unordered_multimap<K, V> |
- |
- |
- |
bitset<N> |
- |
- |
## Benchmark
[//]: # (No deletion!!! Start of Replace Section)
deque
test name | time taken (ms) | executions per sec | sample deviation |
---|
1,000,000 push | 18.17 | 55.04 | 0.00 |
1,000,000 push & pop | 26.06 | 38.37 | 0.00 |
1,000,000 push & shift | 26.79 | 37.32 | 0.00 |
1,000,000 unshift & shift | 25.13 | 39.80 | 0.00 |
[//]: # (No deletion!!! End of Replace Section)
## Codebase design
### Adhere to ES6 and ESNext standard naming conventions for APIs.
Standardize API conventions by using 'add' and 'delete' for element manipulation methods in all data structures.
Opt for concise and clear method names, avoiding excessive length while ensuring explicit intent.
### Object-oriented programming(OOP)
By strictly adhering to object-oriented design (BinaryTree -> BST -> AVLTree -> TreeMultimap), you can seamlessly
inherit the existing data structures to implement the customized ones you need. Object-oriented design stands as the
optimal approach to data structure design.