mirror of
https://github.com/zrwusa/data-structure-typed.git
synced 2025-05-16 06:13:32 +00:00
docs:Sequential Organization
This commit is contained in:
parent
baf1be4035
commit
a9c556fce3
5 changed files with 344 additions and 226 deletions
402
README.md
402
README.md
|
@ -10,7 +10,7 @@
|
|||
|
||||
[//]: # ()
|
||||
|
||||
<p><a href="https://github.com/zrwusa/data-structure-typed/blob/main/README.md">English</a> | <a href="https://github.com/zrwusa/data-structure-typed/blob/main/README_zh-CN.md">简体中文</a></p>
|
||||
[//]: # (<p><a href="https://github.com/zrwusa/data-structure-typed/blob/main/README.md">English</a> | <a href="https://github.com/zrwusa/data-structure-typed/blob/main/README_zh-CN.md">简体中文</a></p>)
|
||||
|
||||
## Why
|
||||
|
||||
|
@ -91,17 +91,6 @@ Heap, Binary Tree, RedBlack Tree, Linked List, Deque, Trie, Directed Graph, Undi
|
|||
|
||||
## Installation and Usage
|
||||
|
||||
Now you can use it in Node.js and browser environments
|
||||
|
||||
CommonJS:**`require export.modules =`**
|
||||
|
||||
ESModule: **`import export`**
|
||||
|
||||
Typescript: **`import export`**
|
||||
|
||||
UMD: **`var Deque = dataStructureTyped.Deque`**
|
||||
|
||||
|
||||
### npm
|
||||
|
||||
```bash
|
||||
|
@ -122,51 +111,12 @@ import {
|
|||
} from 'data-structure-typed';
|
||||
```
|
||||
|
||||
### CDN
|
||||
|
||||
Copy the line below into the head tag in an HTML document.
|
||||
|
||||
#### development
|
||||
|
||||
```html
|
||||
<script src='https://cdn.jsdelivr.net/npm/data-structure-typed/dist/umd/data-structure-typed.js'></script>
|
||||
```
|
||||
|
||||
#### production
|
||||
|
||||
```html
|
||||
<script src='https://cdn.jsdelivr.net/npm/data-structure-typed/dist/umd/data-structure-typed.min.js'></script>
|
||||
```
|
||||
|
||||
Copy the code below into the script tag of your HTML, and you're good to go with your development.
|
||||
|
||||
```js
|
||||
const {Heap} = dataStructureTyped;
|
||||
const {
|
||||
BinaryTree, Graph, Queue, Stack, PriorityQueue, BST, Trie, DoublyLinkedList,
|
||||
AVLTree, MinHeap, SinglyLinkedList, DirectedGraph, TreeMultimap,
|
||||
DirectedVertex, AVLTreeNode
|
||||
} = dataStructureTyped;
|
||||
```
|
||||
|
||||
## Vivid Examples
|
||||
|
||||
### Binary Tree
|
||||
|
||||
[Try it out](https://vivid-algorithm.vercel.app/), or you can run your own code using
|
||||
our [visual tool](https://github.com/zrwusa/vivid-algorithm)
|
||||
|
||||
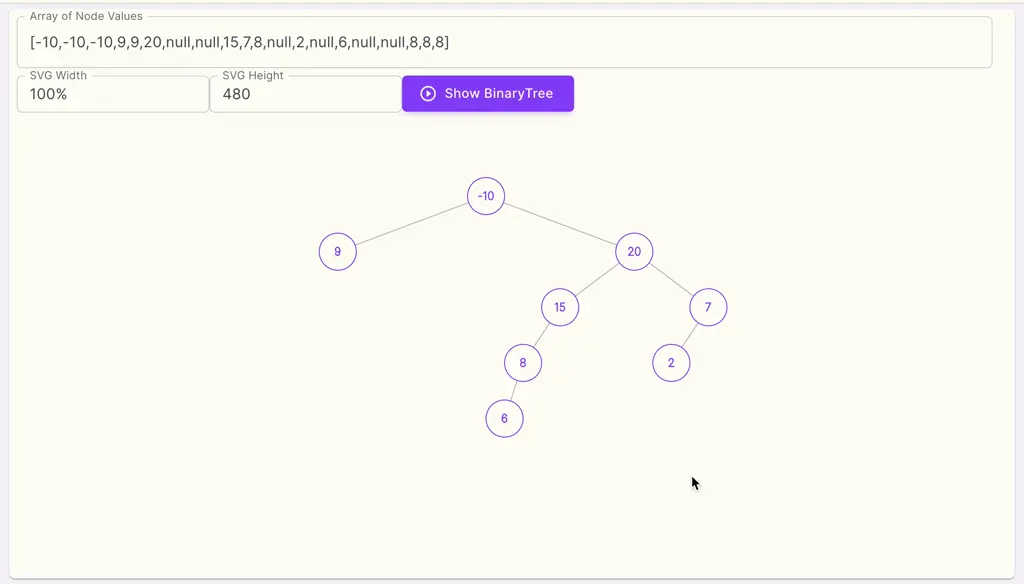
|
||||
|
||||
### Binary Tree DFS
|
||||
|
||||
[Try it out](https://vivid-algorithm.vercel.app/)
|
||||
|
||||
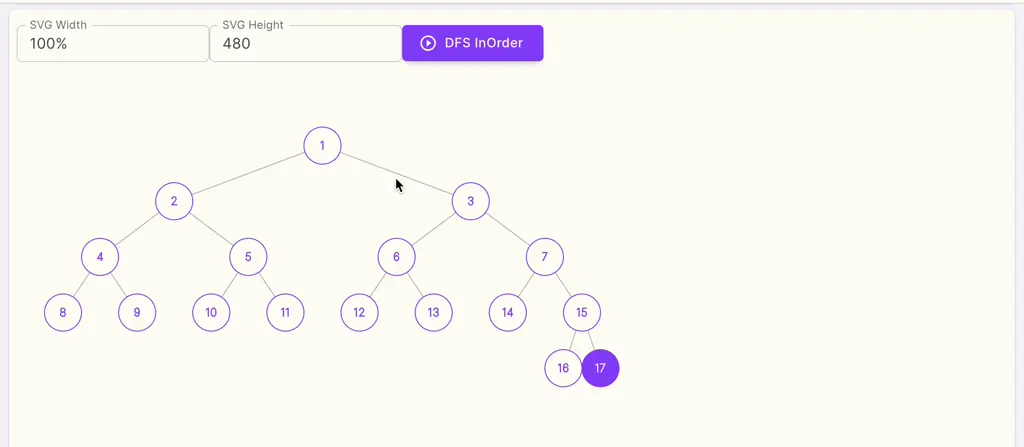
|
||||
|
||||
### AVL Tree
|
||||
|
||||
[Try it out](https://vivid-algorithm.vercel.app/)
|
||||
[Try it out](https://vivid-algorithm.vercel.app/), or you can run your own code using
|
||||
our [visual tool](https://github.com/zrwusa/vivid-algorithm)
|
||||
|
||||
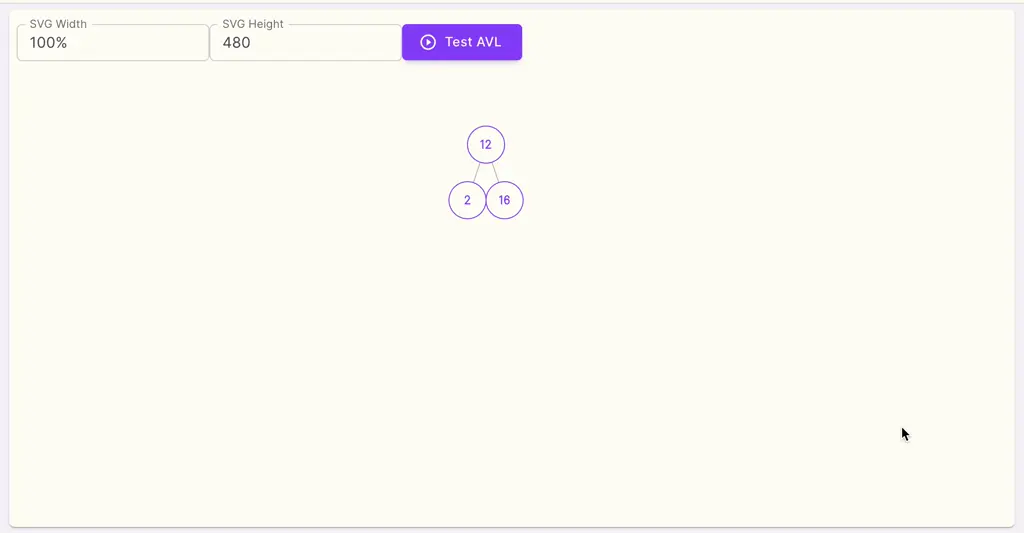
|
||||
|
||||
|
@ -176,12 +126,6 @@ our [visual tool](https://github.com/zrwusa/vivid-algorithm)
|
|||
|
||||
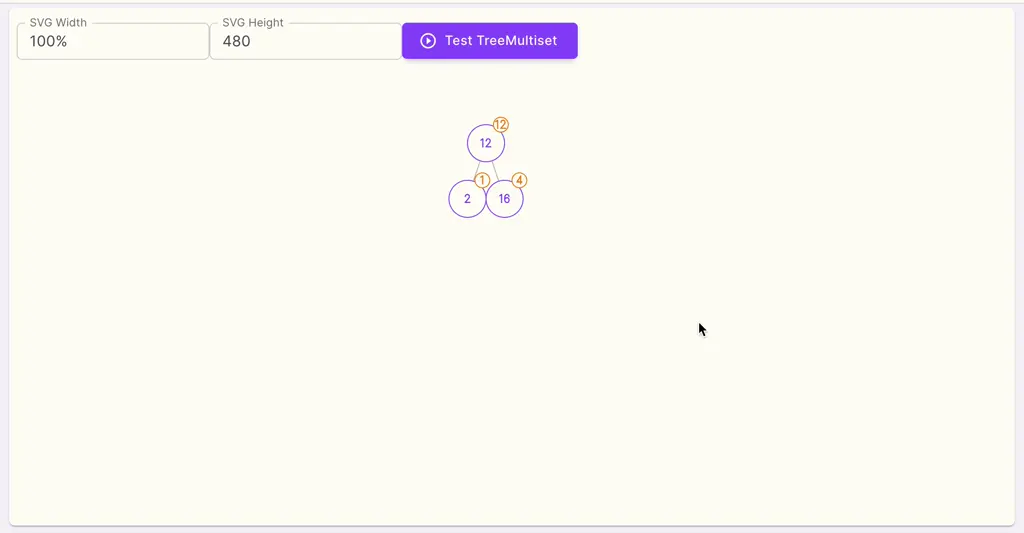
|
||||
|
||||
### Matrix
|
||||
|
||||
[Try it out](https://vivid-algorithm.vercel.app/algorithm/graph/)
|
||||
|
||||
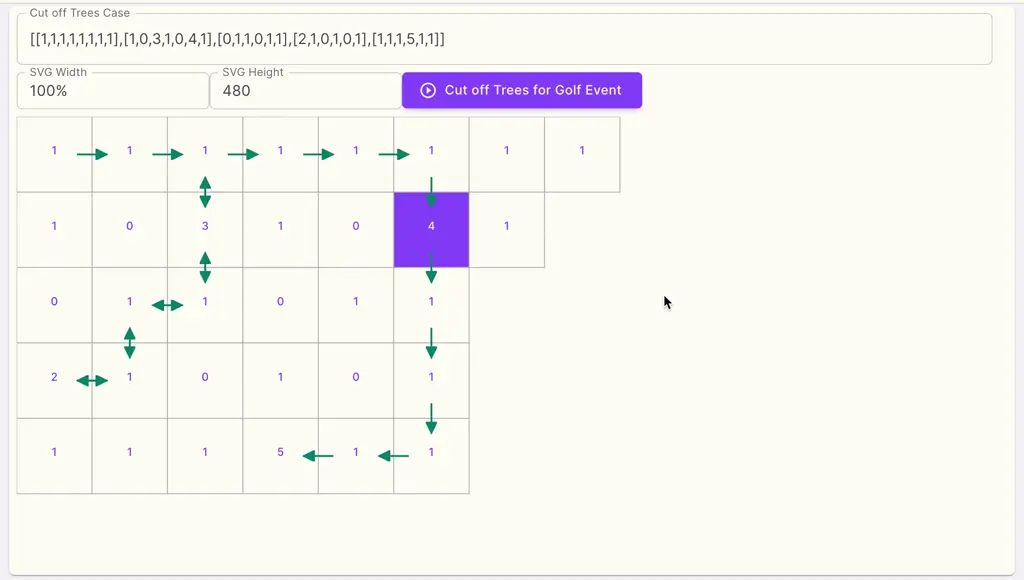
|
||||
|
||||
### Directed Graph
|
||||
|
||||
[Try it out](https://vivid-algorithm.vercel.app/algorithm/graph/)
|
||||
|
@ -242,6 +186,156 @@ rbTree.print()
|
|||
// 13 16
|
||||
```
|
||||
|
||||
### Free conversion between data structures.
|
||||
|
||||
```js
|
||||
const orgArr = [6, 1, 2, 7, 5, 3, 4, 9, 8];
|
||||
const orgStrArr = ["trie", "trial", "trick", "trip", "tree", "trend", "triangle", "track", "trace", "transmit"];
|
||||
const entries = [[6, "6"], [1, "1"], [2, "2"], [7, "7"], [5, "5"], [3, "3"], [4, "4"], [9, "9"], [8, "8"]];
|
||||
|
||||
const queue = new Queue(orgArr);
|
||||
queue.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const deque = new Deque(orgArr);
|
||||
deque.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const sList = new SinglyLinkedList(orgArr);
|
||||
sList.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const dList = new DoublyLinkedList(orgArr);
|
||||
dList.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const stack = new Stack(orgArr);
|
||||
stack.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const minHeap = new MinHeap(orgArr);
|
||||
minHeap.print();
|
||||
// [1, 5, 2, 7, 6, 3, 4, 9, 8]
|
||||
|
||||
const maxPQ = new MaxPriorityQueue(orgArr);
|
||||
maxPQ.print();
|
||||
// [9, 8, 4, 7, 5, 2, 3, 1, 6]
|
||||
|
||||
const biTree = new BinaryTree(entries);
|
||||
biTree.print();
|
||||
// ___6___
|
||||
// / \
|
||||
// ___1_ _2_
|
||||
// / \ / \
|
||||
// _7_ 5 3 4
|
||||
// / \
|
||||
// 9 8
|
||||
|
||||
const bst = new BST(entries);
|
||||
bst.print();
|
||||
// _____5___
|
||||
// / \
|
||||
// _2_ _7_
|
||||
// / \ / \
|
||||
// 1 3_ 6 8_
|
||||
// \ \
|
||||
// 4 9
|
||||
|
||||
|
||||
const rbTree = new RedBlackTree(entries);
|
||||
rbTree.print();
|
||||
// ___4___
|
||||
// / \
|
||||
// _2_ _6___
|
||||
// / \ / \
|
||||
// 1 3 5 _8_
|
||||
// / \
|
||||
// 7 9
|
||||
|
||||
|
||||
const avl = new AVLTree(entries);
|
||||
avl.print();
|
||||
// ___4___
|
||||
// / \
|
||||
// _2_ _6___
|
||||
// / \ / \
|
||||
// 1 3 5 _8_
|
||||
// / \
|
||||
// 7 9
|
||||
|
||||
const treeMulti = new TreeMultimap(entries);
|
||||
treeMulti.print();
|
||||
// ___4___
|
||||
// / \
|
||||
// _2_ _6___
|
||||
// / \ / \
|
||||
// 1 3 5 _8_
|
||||
// / \
|
||||
// 7 9
|
||||
|
||||
const hm = new HashMap(entries);
|
||||
hm.print()
|
||||
// [[6, "6"], [1, "1"], [2, "2"], [7, "7"], [5, "5"], [3, "3"], [4, "4"], [9, "9"], [8, "8"]]
|
||||
|
||||
const rbTreeH = new RedBlackTree(hm);
|
||||
rbTreeH.print();
|
||||
// ___4___
|
||||
// / \
|
||||
// _2_ _6___
|
||||
// / \ / \
|
||||
// 1 3 5 _8_
|
||||
// / \
|
||||
// 7 9
|
||||
|
||||
const pq = new MinPriorityQueue(orgArr);
|
||||
pq.print();
|
||||
// [1, 5, 2, 7, 6, 3, 4, 9, 8]
|
||||
|
||||
const bst1 = new BST(pq);
|
||||
bst1.print();
|
||||
// _____5___
|
||||
// / \
|
||||
// _2_ _7_
|
||||
// / \ / \
|
||||
// 1 3_ 6 8_
|
||||
// \ \
|
||||
// 4 9
|
||||
|
||||
const dq1 = new Deque(orgArr);
|
||||
dq1.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
const rbTree1 = new RedBlackTree(dq1);
|
||||
rbTree1.print();
|
||||
// _____5___
|
||||
// / \
|
||||
// _2___ _7___
|
||||
// / \ / \
|
||||
// 1 _4 6 _9
|
||||
// / /
|
||||
// 3 8
|
||||
|
||||
|
||||
const trie2 = new Trie(orgStrArr);
|
||||
trie2.print();
|
||||
// ['trie', 'trial', 'triangle', 'trick', 'trip', 'tree', 'trend', 'track', 'trace', 'transmit']
|
||||
const heap2 = new Heap(trie2, { comparator: (a, b) => Number(a) - Number(b) });
|
||||
heap2.print();
|
||||
// ['transmit', 'trace', 'tree', 'trend', 'track', 'trial', 'trip', 'trie', 'trick', 'triangle']
|
||||
const dq2 = new Deque(heap2);
|
||||
dq2.print();
|
||||
// ['transmit', 'trace', 'tree', 'trend', 'track', 'trial', 'trip', 'trie', 'trick', 'triangle']
|
||||
const entries2 = dq2.map((el, i) => [i, el]);
|
||||
const avl2 = new AVLTree(entries2);
|
||||
avl2.print();
|
||||
// ___3_______
|
||||
// / \
|
||||
// _1_ ___7_
|
||||
// / \ / \
|
||||
// 0 2 _5_ 8_
|
||||
// / \ \
|
||||
// 4 6 9
|
||||
```
|
||||
|
||||
### Binary Search Tree (BST) snippet
|
||||
|
||||
```ts
|
||||
|
@ -364,156 +458,6 @@ Array.from(dijkstraResult?.seen ?? []).map(vertex => vertex.key) // ['A', 'B', '
|
|||
|
||||
```
|
||||
|
||||
### Free conversion between data structures.
|
||||
|
||||
```js
|
||||
const orgArr = [6, 1, 2, 7, 5, 3, 4, 9, 8];
|
||||
const orgStrArr = ["trie", "trial", "trick", "trip", "tree", "trend", "triangle", "track", "trace", "transmit"];
|
||||
const entries = [[6, 6], [1, 1], [2, 2], [7, 7], [5, 5], [3, 3], [4, 4], [9, 9], [8, 8]];
|
||||
|
||||
const queue = new Queue(orgArr);
|
||||
queue.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const deque = new Deque(orgArr);
|
||||
deque.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const sList = new SinglyLinkedList(orgArr);
|
||||
sList.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const dList = new DoublyLinkedList(orgArr);
|
||||
dList.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const stack = new Stack(orgArr);
|
||||
stack.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
|
||||
const minHeap = new MinHeap(orgArr);
|
||||
minHeap.print();
|
||||
// [1, 5, 2, 7, 6, 3, 4, 9, 8]
|
||||
|
||||
const maxPQ = new MaxPriorityQueue(orgArr);
|
||||
maxPQ.print();
|
||||
// [9, 8, 4, 7, 5, 2, 3, 1, 6]
|
||||
|
||||
const biTree = new BinaryTree(entries);
|
||||
biTree.print();
|
||||
// ___6___
|
||||
// / \
|
||||
// ___1_ _2_
|
||||
// / \ / \
|
||||
// _7_ 5 3 4
|
||||
// / \
|
||||
// 9 8
|
||||
|
||||
const bst = new BST(entries);
|
||||
bst.print();
|
||||
// _____5___
|
||||
// / \
|
||||
// _2_ _7_
|
||||
// / \ / \
|
||||
// 1 3_ 6 8_
|
||||
// \ \
|
||||
// 4 9
|
||||
|
||||
|
||||
const rbTree = new RedBlackTree(entries);
|
||||
rbTree.print();
|
||||
// ___4___
|
||||
// / \
|
||||
// _2_ _6___
|
||||
// / \ / \
|
||||
// 1 3 5 _8_
|
||||
// / \
|
||||
// 7 9
|
||||
|
||||
|
||||
const avl = new AVLTree(entries);
|
||||
avl.print();
|
||||
// ___4___
|
||||
// / \
|
||||
// _2_ _6___
|
||||
// / \ / \
|
||||
// 1 3 5 _8_
|
||||
// / \
|
||||
// 7 9
|
||||
|
||||
const treeMulti = new TreeMultimap(entries);
|
||||
treeMulti.print();
|
||||
// ___4___
|
||||
// / \
|
||||
// _2_ _6___
|
||||
// / \ / \
|
||||
// 1 3 5 _8_
|
||||
// / \
|
||||
// 7 9
|
||||
|
||||
const hm = new HashMap(entries);
|
||||
hm.print()
|
||||
// [[6, 6], [1, 1], [2, 2], [7, 7], [5, 5], [3, 3], [4, 4], [9, 9], [8, 8]]
|
||||
|
||||
const rbTreeH = new RedBlackTree(hm);
|
||||
rbTreeH.print();
|
||||
// ___4___
|
||||
// / \
|
||||
// _2_ _6___
|
||||
// / \ / \
|
||||
// 1 3 5 _8_
|
||||
// / \
|
||||
// 7 9
|
||||
|
||||
const pq = new MinPriorityQueue(orgArr);
|
||||
pq.print();
|
||||
// [1, 5, 2, 7, 6, 3, 4, 9, 8]
|
||||
|
||||
const bst1 = new BST(pq);
|
||||
bst1.print();
|
||||
// _____5___
|
||||
// / \
|
||||
// _2_ _7_
|
||||
// / \ / \
|
||||
// 1 3_ 6 8_
|
||||
// \ \
|
||||
// 4 9
|
||||
|
||||
const dq1 = new Deque(orgArr);
|
||||
dq1.print();
|
||||
// [6, 1, 2, 7, 5, 3, 4, 9, 8]
|
||||
const rbTree1 = new RedBlackTree(dq1);
|
||||
rbTree1.print();
|
||||
// _____5___
|
||||
// / \
|
||||
// _2___ _7___
|
||||
// / \ / \
|
||||
// 1 _4 6 _9
|
||||
// / /
|
||||
// 3 8
|
||||
|
||||
|
||||
const trie2 = new Trie(orgStrArr);
|
||||
trie2.print();
|
||||
// ['trie', 'trial', 'triangle', 'trick', 'trip', 'tree', 'trend', 'track', 'trace', 'transmit']
|
||||
const heap2 = new Heap(trie2, { comparator: (a, b) => Number(a) - Number(b) });
|
||||
heap2.print();
|
||||
// ['transmit', 'trace', 'tree', 'trend', 'track', 'trial', 'trip', 'trie', 'trick', 'triangle']
|
||||
const dq2 = new Deque(heap2);
|
||||
dq2.print();
|
||||
// ['transmit', 'trace', 'tree', 'trend', 'track', 'trial', 'trip', 'trie', 'trick', 'triangle']
|
||||
const entries2 = dq2.map((el, i) => [i, el]);
|
||||
const avl2 = new AVLTree(entries2);
|
||||
avl2.print();
|
||||
// ___3_______
|
||||
// / \
|
||||
// _1_ ___7_
|
||||
// / \ / \
|
||||
// 0 2 _5_ 8_
|
||||
// / \ \
|
||||
// 4 6 9
|
||||
```
|
||||
|
||||
## API docs & Examples
|
||||
|
||||
[API Docs](https://data-structure-typed-docs.vercel.app)
|
||||
|
@ -1027,3 +971,43 @@ We strictly adhere to computer science theory and software development standards
|
|||
</div>
|
||||
|
||||
[//]: # (No deletion!!! End of Replace Section)
|
||||
|
||||
|
||||
|
||||
## supported module system
|
||||
Now you can use it in Node.js and browser environments
|
||||
|
||||
CommonJS:**`require export.modules =`**
|
||||
|
||||
ESModule: **`import export`**
|
||||
|
||||
Typescript: **`import export`**
|
||||
|
||||
UMD: **`var Deque = dataStructureTyped.Deque`**
|
||||
|
||||
### CDN
|
||||
|
||||
Copy the line below into the head tag in an HTML document.
|
||||
|
||||
#### development
|
||||
|
||||
```html
|
||||
<script src='https://cdn.jsdelivr.net/npm/data-structure-typed/dist/umd/data-structure-typed.js'></script>
|
||||
```
|
||||
|
||||
#### production
|
||||
|
||||
```html
|
||||
<script src='https://cdn.jsdelivr.net/npm/data-structure-typed/dist/umd/data-structure-typed.min.js'></script>
|
||||
```
|
||||
|
||||
Copy the code below into the script tag of your HTML, and you're good to go with your development.
|
||||
|
||||
```js
|
||||
const {Heap} = dataStructureTyped;
|
||||
const {
|
||||
BinaryTree, Graph, Queue, Stack, PriorityQueue, BST, Trie, DoublyLinkedList,
|
||||
AVLTree, MinHeap, SinglyLinkedList, DirectedGraph, TreeMultimap,
|
||||
DirectedVertex, AVLTreeNode
|
||||
} = dataStructureTyped;
|
||||
```
|
|
@ -622,6 +622,6 @@ describe('LinkedHashMap setMany, keys, values', () => {
|
|||
});
|
||||
|
||||
test('print', () => {
|
||||
hm.print();
|
||||
// hm.print();
|
||||
});
|
||||
});
|
||||
|
|
|
@ -164,13 +164,14 @@ describe('Deque - Utility Operations', () => {
|
|||
});
|
||||
|
||||
test('print should print the deque elements', () => {
|
||||
const consoleSpy = jest.spyOn(console, 'log');
|
||||
deque.push(1);
|
||||
deque.push(2);
|
||||
deque.print();
|
||||
expect(consoleSpy).toHaveBeenCalledWith([1, 2]);
|
||||
// const consoleSpy = jest.spyOn(console, 'log');
|
||||
// deque.push(1);
|
||||
// deque.push(2);
|
||||
// deque.print();
|
||||
// expect(consoleSpy).toHaveBeenCalledWith([1, 2]);
|
||||
});
|
||||
});
|
||||
|
||||
describe('Deque - Additional Operations', () => {
|
||||
let deque: Deque<number>;
|
||||
|
||||
|
|
|
@ -172,7 +172,7 @@ describe('Queue - Additional Methods', () => {
|
|||
test('print should not throw any errors', () => {
|
||||
expect(() => {
|
||||
queue.push(1);
|
||||
queue.print();
|
||||
// queue.print();
|
||||
}).not.toThrow();
|
||||
});
|
||||
});
|
||||
|
|
|
@ -33,91 +33,155 @@ const orgStrArr: string[] = [
|
|||
'trace',
|
||||
'transmit'
|
||||
];
|
||||
const entries: [number, number][] = [
|
||||
[6, 6],
|
||||
[1, 1],
|
||||
[2, 2],
|
||||
[7, 7],
|
||||
[5, 5],
|
||||
[3, 3],
|
||||
[4, 4],
|
||||
[9, 9],
|
||||
[8, 8]
|
||||
const entries: [number, string][] = [
|
||||
[6, '6'],
|
||||
[1, '1'],
|
||||
[2, '2'],
|
||||
[7, '7'],
|
||||
[5, '5'],
|
||||
[3, '3'],
|
||||
[4, '4'],
|
||||
[9, '9'],
|
||||
[8, '8']
|
||||
];
|
||||
|
||||
describe('conversions', () => {
|
||||
it('Array to Queue', () => {
|
||||
const q = new Queue<number>(orgArr);
|
||||
isDebug && q.print();
|
||||
expect([...q]).toEqual([6, 1, 2, 7, 5, 3, 4, 9, 8]);
|
||||
});
|
||||
|
||||
it('Array to Deque', () => {
|
||||
const dq = new Deque<number>(orgArr);
|
||||
isDebug && dq.print();
|
||||
expect([...dq]).toEqual([6, 1, 2, 7, 5, 3, 4, 9, 8]);
|
||||
});
|
||||
|
||||
it('Array to SinglyLinkedList', () => {
|
||||
const sl = new SinglyLinkedList<number>(orgArr);
|
||||
isDebug && sl.print();
|
||||
expect([...sl]).toEqual([6, 1, 2, 7, 5, 3, 4, 9, 8]);
|
||||
});
|
||||
|
||||
it('Array to DoublyLinkedList', () => {
|
||||
const dl = new DoublyLinkedList<number>(orgArr);
|
||||
isDebug && dl.print();
|
||||
expect([...dl]).toEqual([6, 1, 2, 7, 5, 3, 4, 9, 8]);
|
||||
});
|
||||
|
||||
it('Array to Stack', () => {
|
||||
const stack = new Stack<number>(orgArr);
|
||||
isDebug && stack.print();
|
||||
expect([...stack]).toEqual([6, 1, 2, 7, 5, 3, 4, 9, 8]);
|
||||
});
|
||||
|
||||
it('Array to MinHeap', () => {
|
||||
const minHeap = new MinHeap<number>(orgArr);
|
||||
isDebug && minHeap.print();
|
||||
expect([...minHeap]).toEqual([1, 5, 2, 7, 6, 3, 4, 9, 8]);
|
||||
});
|
||||
|
||||
it('Array to MaxHeap', () => {
|
||||
const maxHeap = new MaxHeap<number>(orgArr);
|
||||
isDebug && maxHeap.print();
|
||||
expect([...maxHeap]).toEqual([9, 8, 4, 7, 5, 2, 3, 1, 6]);
|
||||
});
|
||||
|
||||
it('Array to MinPriorityQueue', () => {
|
||||
const minPQ = new MinPriorityQueue<number>(orgArr);
|
||||
isDebug && minPQ.print();
|
||||
expect([...minPQ]).toEqual([1, 5, 2, 7, 6, 3, 4, 9, 8]);
|
||||
});
|
||||
|
||||
it('Array to MaxPriorityQueue', () => {
|
||||
const maxPQ = new MaxPriorityQueue<number>(orgArr);
|
||||
isDebug && maxPQ.print();
|
||||
expect([...maxPQ]).toEqual([9, 8, 4, 7, 5, 2, 3, 1, 6]);
|
||||
});
|
||||
|
||||
it('Entry Array to BinaryTree', () => {
|
||||
const biTree = new BinaryTree<number>(entries);
|
||||
isDebug && biTree.print();
|
||||
expect([...biTree]).toEqual([
|
||||
[9, '9'],
|
||||
[7, '7'],
|
||||
[8, '8'],
|
||||
[1, '1'],
|
||||
[5, '5'],
|
||||
[6, '6'],
|
||||
[3, '3'],
|
||||
[2, '2'],
|
||||
[4, '4']
|
||||
]);
|
||||
});
|
||||
|
||||
it('Entry Array to BST', () => {
|
||||
const bst = new BST<number>(entries);
|
||||
expect(bst.size).toBe(9);
|
||||
isDebug && bst.print();
|
||||
expect([...bst]).toEqual([
|
||||
[1, '1'],
|
||||
[2, '2'],
|
||||
[3, '3'],
|
||||
[4, '4'],
|
||||
[5, '5'],
|
||||
[6, '6'],
|
||||
[7, '7'],
|
||||
[8, '8'],
|
||||
[9, '9']
|
||||
]);
|
||||
});
|
||||
|
||||
it('Entry Array to RedBlackTree', () => {
|
||||
const rbTree = new RedBlackTree<number>(entries);
|
||||
expect(rbTree.size).toBe(9);
|
||||
isDebug && rbTree.print();
|
||||
expect([...rbTree]).toEqual([
|
||||
[1, '1'],
|
||||
[2, '2'],
|
||||
[3, '3'],
|
||||
[4, '4'],
|
||||
[5, '5'],
|
||||
[6, '6'],
|
||||
[7, '7'],
|
||||
[8, '8'],
|
||||
[9, '9']
|
||||
]);
|
||||
});
|
||||
|
||||
it('Entry Array to AVLTree', () => {
|
||||
const avl = new AVLTree<number>(entries);
|
||||
expect(avl.size).toBe(9);
|
||||
isDebug && avl.print();
|
||||
expect([...avl]).toEqual([
|
||||
[1, '1'],
|
||||
[2, '2'],
|
||||
[3, '3'],
|
||||
[4, '4'],
|
||||
[5, '5'],
|
||||
[6, '6'],
|
||||
[7, '7'],
|
||||
[8, '8'],
|
||||
[9, '9']
|
||||
]);
|
||||
});
|
||||
|
||||
it('Entry Array to TreeMultimap', () => {
|
||||
const treeMulti = new TreeMultimap<number>(entries);
|
||||
expect(treeMulti.size).toBe(9);
|
||||
isDebug && treeMulti.print();
|
||||
expect([...treeMulti]).toEqual([
|
||||
[1, '1'],
|
||||
[2, '2'],
|
||||
[3, '3'],
|
||||
[4, '4'],
|
||||
[5, '5'],
|
||||
[6, '6'],
|
||||
[7, '7'],
|
||||
[8, '8'],
|
||||
[9, '9']
|
||||
]);
|
||||
});
|
||||
|
||||
it('HashMap to RedBlackTree', () => {
|
||||
|
@ -126,6 +190,17 @@ describe('conversions', () => {
|
|||
const rbTree = new RedBlackTree<number>(hm);
|
||||
expect(rbTree.size).toBe(9);
|
||||
isDebug && rbTree.print();
|
||||
expect([...rbTree]).toEqual([
|
||||
[1, '1'],
|
||||
[2, '2'],
|
||||
[3, '3'],
|
||||
[4, '4'],
|
||||
[5, '5'],
|
||||
[6, '6'],
|
||||
[7, '7'],
|
||||
[8, '8'],
|
||||
[9, '9']
|
||||
]);
|
||||
});
|
||||
|
||||
it('PriorityQueue to BST', () => {
|
||||
|
@ -134,6 +209,17 @@ describe('conversions', () => {
|
|||
const bst = new BST<number>(pq);
|
||||
expect(bst.size).toBe(9);
|
||||
isDebug && bst.print();
|
||||
expect([...bst]).toEqual([
|
||||
[1, undefined],
|
||||
[2, undefined],
|
||||
[3, undefined],
|
||||
[4, undefined],
|
||||
[5, undefined],
|
||||
[6, undefined],
|
||||
[7, undefined],
|
||||
[8, undefined],
|
||||
[9, undefined]
|
||||
]);
|
||||
});
|
||||
|
||||
it('Deque to RedBlackTree', () => {
|
||||
|
@ -142,6 +228,17 @@ describe('conversions', () => {
|
|||
const rbTree = new RedBlackTree<number>(dq);
|
||||
expect(rbTree.size).toBe(9);
|
||||
isDebug && rbTree.print();
|
||||
expect([...rbTree]).toEqual([
|
||||
[1, undefined],
|
||||
[2, undefined],
|
||||
[3, undefined],
|
||||
[4, undefined],
|
||||
[5, undefined],
|
||||
[6, undefined],
|
||||
[7, undefined],
|
||||
[8, undefined],
|
||||
[9, undefined]
|
||||
]);
|
||||
});
|
||||
|
||||
it('Trie to Heap to Deque', () => {
|
||||
|
@ -151,12 +248,48 @@ describe('conversions', () => {
|
|||
const heap = new Heap<string>(trie, { comparator: (a, b) => Number(a) - Number(b) });
|
||||
expect(heap.size).toBe(10);
|
||||
isDebug && heap.print();
|
||||
expect([...heap]).toEqual([
|
||||
'transmit',
|
||||
'trace',
|
||||
'tree',
|
||||
'trend',
|
||||
'track',
|
||||
'trial',
|
||||
'trip',
|
||||
'trie',
|
||||
'trick',
|
||||
'triangle'
|
||||
]);
|
||||
const dq = new Deque<string>(heap);
|
||||
expect(dq.size).toBe(10);
|
||||
isDebug && dq.print();
|
||||
expect([...dq]).toEqual([
|
||||
'transmit',
|
||||
'trace',
|
||||
'tree',
|
||||
'trend',
|
||||
'track',
|
||||
'trial',
|
||||
'trip',
|
||||
'trie',
|
||||
'trick',
|
||||
'triangle'
|
||||
]);
|
||||
const entries = dq.map((el, i) => <[number, string]>[i, el]);
|
||||
const avl = new AVLTree<number, string>(entries);
|
||||
expect(avl.size).toBe(10);
|
||||
isDebug && avl.print();
|
||||
expect([...avl]).toEqual([
|
||||
[0, 'transmit'],
|
||||
[1, 'trace'],
|
||||
[2, 'tree'],
|
||||
[3, 'trend'],
|
||||
[4, 'track'],
|
||||
[5, 'trial'],
|
||||
[6, 'trip'],
|
||||
[7, 'trie'],
|
||||
[8, 'trick'],
|
||||
[9, 'triangle']
|
||||
]);
|
||||
});
|
||||
});
|
||||
|
|
Loading…
Add table
Reference in a new issue