diff --git a/CHANGELOG.md b/CHANGELOG.md index 711153b..725a3b2 100644 --- a/CHANGELOG.md +++ b/CHANGELOG.md @@ -8,7 +8,7 @@ All notable changes to this project will be documented in this file. - [Semantic Versioning](https://semver.org/spec/v2.0.0.html) - [`auto-changelog`](https://github.com/CookPete/auto-changelog) -## [v1.46.6](https://github.com/zrwusa/data-structure-typed/compare/v1.35.0...main) (upcoming) +## [v1.46.7](https://github.com/zrwusa/data-structure-typed/compare/v1.35.0...main) (upcoming) ### Changes diff --git a/README.md b/README.md index 8f185a5..b98751d 100644 --- a/README.md +++ b/README.md @@ -11,7 +11,7 @@ Data Structures of Javascript & TypeScript. -Do you envy C++ with [STL](), Python with [collections](), and Java with [java.util]() ? Well, no need to envy anymore! JavaScript and TypeScript now have [data-structure-typed](). +Do you envy C++ with [STL]() (std::), Python with [collections](), and Java with [java.util]() ? Well, no need to envy anymore! JavaScript and TypeScript now have [data-structure-typed](). Now you can use this library in Node.js and browser environments in CommonJS(require export.modules = ), ESModule(import export), Typescript(import export), UMD(var Queue = dataStructureTyped.Queue) @@ -24,7 +24,6 @@ Now you can use this library in Node.js and browser environments in CommonJS(req [//]: # () - ## Installation and Usage ### npm @@ -117,8 +116,6 @@ const { 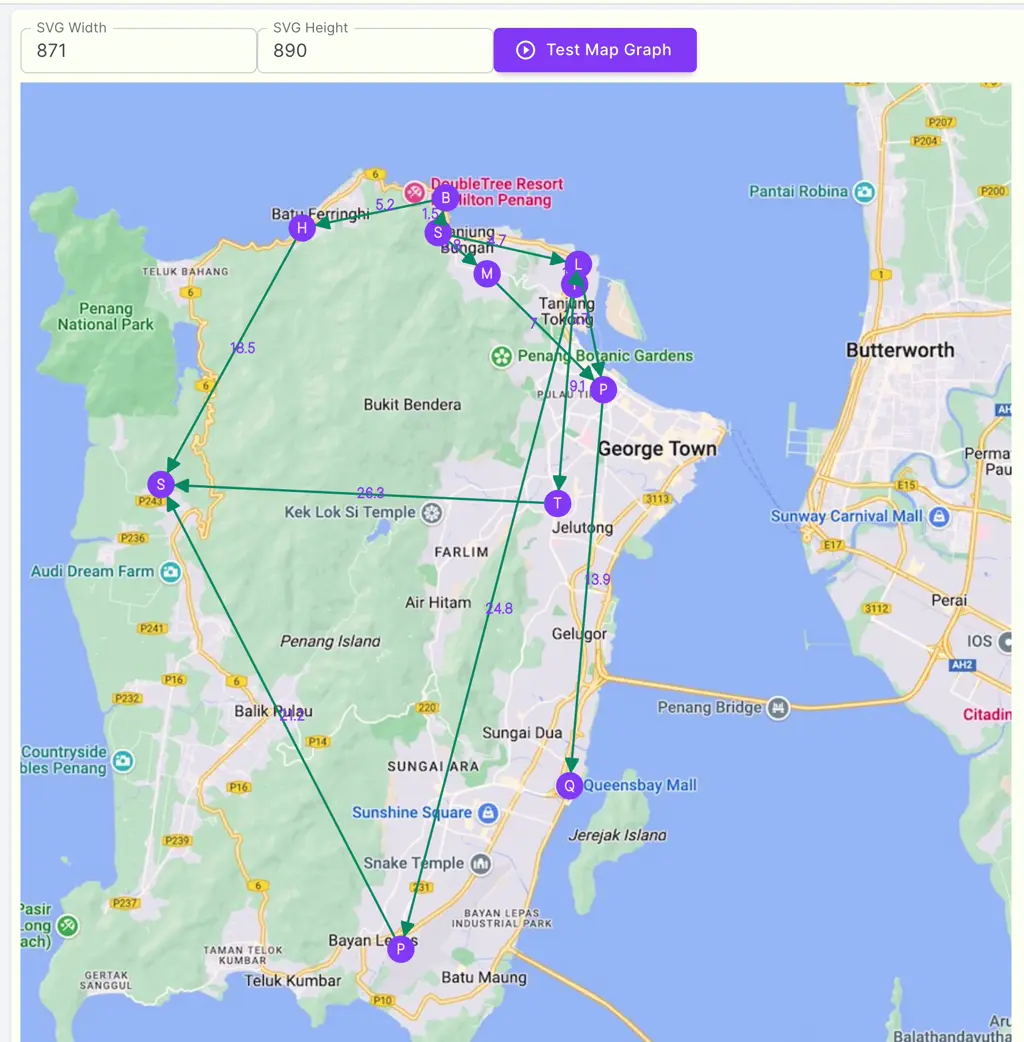 - - ## Code Snippets ### Binary Search Tree (BST) snippet @@ -275,107 +272,6 @@ const dijkstraResult = graph.dijkstra('A'); Array.from(dijkstraResult?.seen ?? []).map(vertex => vertex.key) // ['A', 'B', 'D'] ``` -## Built-in classic algorithms - -
Algorithm | -Function Description | -Iteration Type | -
---|---|---|
Binary Tree DFS | -Traverse a binary tree in a depth-first manner, starting from the root node, first visiting the left subtree, - and then the right subtree, using recursion. - | -Recursion + Iteration | -
Binary Tree BFS | -Traverse a binary tree in a breadth-first manner, starting from the root node, visiting nodes level by level - from left to right. - | -Iteration | -
Graph DFS | -Traverse a graph in a depth-first manner, starting from a given node, exploring along one path as deeply as - possible, and backtracking to explore other paths. Used for finding connected components, paths, etc. - | -Recursion + Iteration | -
Binary Tree Morris | -Morris traversal is an in-order traversal algorithm for binary trees with O(1) space complexity. It allows tree - traversal without additional stack or recursion. - | -Iteration | -
Graph BFS | -Traverse a graph in a breadth-first manner, starting from a given node, first visiting nodes directly connected - to the starting node, and then expanding level by level. Used for finding shortest paths, etc. - | -Recursion + Iteration | -
Graph Tarjan's Algorithm | -Find strongly connected components in a graph, typically implemented using depth-first search. | -Recursion | -
Graph Bellman-Ford Algorithm | -Finding the shortest paths from a single source, can handle negative weight edges | -Iteration | -
Graph Dijkstra's Algorithm | -Finding the shortest paths from a single source, cannot handle negative weight edges | -Iteration | -
Graph Floyd-Warshall Algorithm | -Finding the shortest paths between all pairs of nodes | -Iteration | -
Graph getCycles | -Find all cycles in a graph or detect the presence of cycles. | -Recursion | -
Graph getCutVertexes | -Find cut vertices in a graph, which are nodes that, when removed, increase the number of connected components in - the graph. - | -Recursion | -
Graph getSCCs | -Find strongly connected components in a graph, which are subgraphs where any two nodes can reach each other. - | -Recursion | -
Graph getBridges | -Find bridges in a graph, which are edges that, when removed, increase the number of connected components in the - graph. - | -Recursion | -
Graph topologicalSort | -Perform topological sorting on a directed acyclic graph (DAG) to find a linear order of nodes such that all - directed edges go from earlier nodes to later nodes. - | -Recursion | -
Algorithm | +Function Description | +Iteration Type | +
---|---|---|
Binary Tree DFS | +Traverse a binary tree in a depth-first manner, starting from the root node, first visiting the left subtree, + and then the right subtree, using recursion. + | +Recursion + Iteration | +
Binary Tree BFS | +Traverse a binary tree in a breadth-first manner, starting from the root node, visiting nodes level by level + from left to right. + | +Iteration | +
Graph DFS | +Traverse a graph in a depth-first manner, starting from a given node, exploring along one path as deeply as + possible, and backtracking to explore other paths. Used for finding connected components, paths, etc. + | +Recursion + Iteration | +
Binary Tree Morris | +Morris traversal is an in-order traversal algorithm for binary trees with O(1) space complexity. It allows tree + traversal without additional stack or recursion. + | +Iteration | +
Graph BFS | +Traverse a graph in a breadth-first manner, starting from a given node, first visiting nodes directly connected + to the starting node, and then expanding level by level. Used for finding shortest paths, etc. + | +Recursion + Iteration | +
Graph Tarjan's Algorithm | +Find strongly connected components in a graph, typically implemented using depth-first search. | +Recursion | +
Graph Bellman-Ford Algorithm | +Finding the shortest paths from a single source, can handle negative weight edges | +Iteration | +
Graph Dijkstra's Algorithm | +Finding the shortest paths from a single source, cannot handle negative weight edges | +Iteration | +
Graph Floyd-Warshall Algorithm | +Finding the shortest paths between all pairs of nodes | +Iteration | +
Graph getCycles | +Find all cycles in a graph or detect the presence of cycles. | +Recursion | +
Graph getCutVertexes | +Find cut vertices in a graph, which are nodes that, when removed, increase the number of connected components in + the graph. + | +Recursion | +
Graph getSCCs | +Find strongly connected components in a graph, which are subgraphs where any two nodes can reach each other. + | +Recursion | +
Graph getBridges | +Find bridges in a graph, which are edges that, when removed, increase the number of connected components in the + graph. + | +Recursion | +
Graph topologicalSort | +Perform topological sorting on a directed acyclic graph (DAG) to find a linear order of nodes such that all + directed edges go from earlier nodes to later nodes. + | +Recursion | +
Data structure software does not involve load issues. |