2023-09-22 03:20:33 +00:00
# Data Structure Typed
2023-11-10 15:50:57 +00:00

2023-11-09 12:48:06 +00:00

2023-10-10 09:22:18 +00:00

2023-11-10 15:50:57 +00:00



2023-10-10 09:22:18 +00:00
2023-11-10 15:50:57 +00:00
[//]: # ( )
2023-10-10 09:22:18 +00:00
2023-10-10 14:18:39 +00:00
Data Structures of Javascript & TypeScript.
2023-11-03 17:21:30 +00:00
Do you envy C++ with [STL](), Python with [collections](), and Java with [java.util]() ? Well, no need to envy anymore! JavaScript and TypeScript now have [data-structure-typed]().
2023-10-10 14:18:39 +00:00
Now you can use this library in Node.js and browser environments in CommonJS(require export.modules = ), ESModule(import export), Typescript(import export), UMD(var Queue = dataStructureTyped.Queue)
2023-09-22 02:40:12 +00:00
2023-10-05 02:37:42 +00:00
[//]: # ( )
[//]: # ( )
[//]: # ( )
[//]: # ( )
2023-09-22 03:20:33 +00:00
## Installation and Usage
2023-09-24 13:31:09 +00:00
2023-08-30 16:50:23 +00:00
### npm
2023-09-24 13:31:09 +00:00
2023-08-30 16:50:23 +00:00
```bash
2023-11-10 15:50:57 +00:00
npm i data-structure-typed
2023-08-30 16:50:23 +00:00
```
2023-09-24 13:31:09 +00:00
2023-08-28 12:04:43 +00:00
### yarn
2023-09-24 13:31:09 +00:00
2023-08-28 12:04:43 +00:00
```bash
2023-09-12 03:10:09 +00:00
yarn add data-structure-typed
2023-08-28 12:04:43 +00:00
```
2023-09-24 13:31:09 +00:00
2023-10-05 02:37:42 +00:00
```js
import {
BinaryTree, Graph, Queue, Stack, PriorityQueue, BST, Trie, DoublyLinkedList,
2023-11-08 01:26:43 +00:00
AVLTree, MinHeap, SinglyLinkedList, DirectedGraph, TreeMultimap,
2023-10-05 02:37:42 +00:00
DirectedVertex, AVLTreeNode
} from 'data-structure-typed';
```
2023-09-09 15:49:53 +00:00
### CDN
2023-09-24 13:31:09 +00:00
2023-11-08 12:25:02 +00:00
Copy the line below into the head tag in an HTML document.
2023-11-13 14:30:59 +00:00
#### development
```html
< script src = 'https://cdn.jsdelivr.net/npm/data-structure-typed/dist/umd/data-structure-typed.js' > < / script >
```
#### production
2023-09-09 15:49:53 +00:00
```html
2023-10-27 14:30:52 +00:00
< script src = 'https://cdn.jsdelivr.net/npm/data-structure-typed/dist/umd/data-structure-typed.min.js' > < / script >
2023-09-09 15:49:53 +00:00
```
2023-09-24 13:31:09 +00:00
2023-11-08 12:25:02 +00:00
Copy the code below into the script tag of your HTML, and you're good to go with your development work.
2023-09-22 03:20:33 +00:00
```js
2023-10-05 02:37:42 +00:00
const {Heap} = dataStructureTyped;
2023-09-24 13:31:09 +00:00
const {
2023-10-05 02:37:42 +00:00
BinaryTree, Graph, Queue, Stack, PriorityQueue, BST, Trie, DoublyLinkedList,
2023-11-08 01:26:43 +00:00
AVLTree, MinHeap, SinglyLinkedList, DirectedGraph, TreeMultimap,
2023-10-05 02:37:42 +00:00
DirectedVertex, AVLTreeNode
2023-09-24 13:31:09 +00:00
} = dataStructureTyped;
2023-09-09 15:49:53 +00:00
```
2023-11-17 02:47:13 +00:00
## Software Engineering Design Standards
< table >
< tr >
< th > Principle< / th >
< th > Description< / th >
< / tr >
< tr >
< td > Practicality< / td >
< td > Follows ES6 and ESNext standards, offering unified and considerate optional parameters, and simplifies method names.< / td >
< / tr >
< tr >
< td > Extensibility< / td >
< td > Adheres to OOP (Object-Oriented Programming) principles, allowing inheritance for all data structures.< / td >
< / tr >
< tr >
< td > Modularization< / td >
< td > Includes data structure modularization and independent NPM packages.< / td >
< / tr >
< tr >
< td > Efficiency< / td >
< td > All methods provide time and space complexity, comparable to native JS performance.< / td >
< / tr >
< tr >
< td > Maintainability< / td >
< td > Follows open-source community development standards, complete documentation, continuous integration, and adheres to TDD (Test-Driven Development) patterns.< / td >
< / tr >
< tr >
< td > Testability< / td >
< td > Automated and customized unit testing, performance testing, and integration testing.< / td >
< / tr >
< tr >
< td > Portability< / td >
< td > Plans for porting to Java, Python, and C++, currently achieved to 80%.< / td >
< / tr >
< tr >
< td > Reusability< / td >
< td > Fully decoupled, minimized side effects, and adheres to OOP.< / td >
< / tr >
< tr >
< td > Security< / td >
2023-11-17 08:11:31 +00:00
< td > Carefully designed security for member variables and methods. Read-write separation. Data structure software does not need to consider other security aspects.< / td >
2023-11-17 02:47:13 +00:00
< / tr >
< tr >
< td > Scalability< / td >
< td > Data structure software does not involve load issues.< / td >
< / tr >
< / table >
## Vivid Examples
### Binary Tree
2023-11-16 13:43:45 +00:00
[Try it out ](https://vivid-algorithm.vercel.app/ ), or you can execute your own code using our [visual tool ](https://github.com/zrwusa/vivid-algorithm )
2023-09-18 14:04:39 +00:00
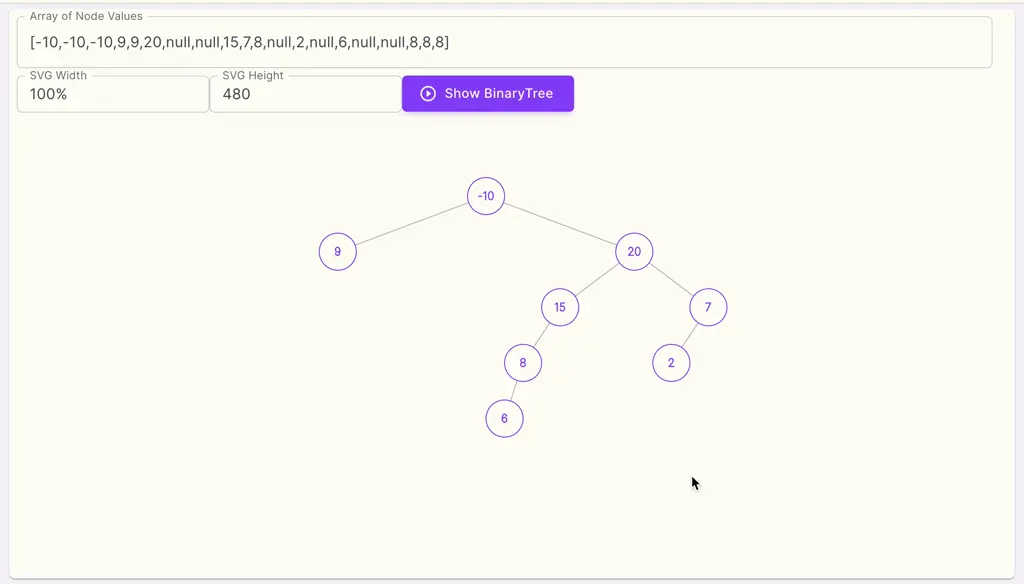
2023-11-16 13:43:45 +00:00
2023-11-17 02:47:13 +00:00
### Binary Tree DFS
2023-11-16 13:43:45 +00:00
[Try it out ](https://vivid-algorithm.vercel.app/ ), or you can execute your own code using our [visual tool ](https://github.com/zrwusa/vivid-algorithm )
2023-09-18 14:04:39 +00:00
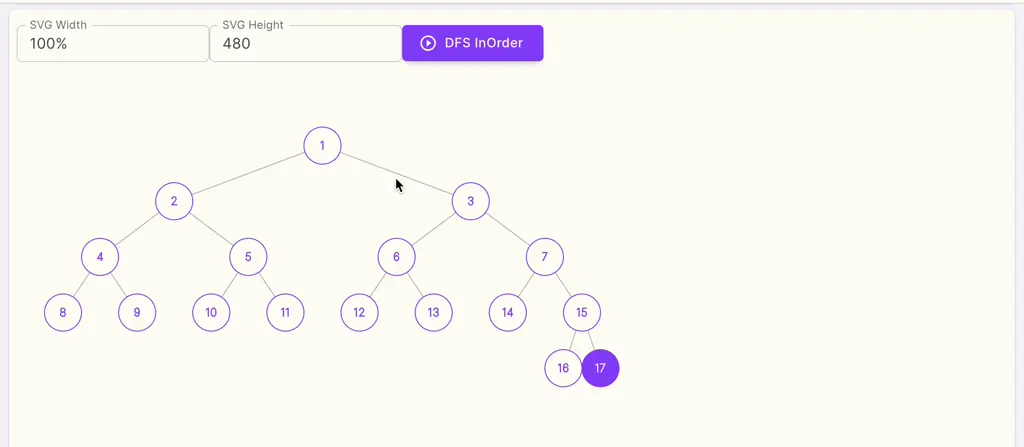
2023-11-16 13:43:45 +00:00
2023-11-17 02:47:13 +00:00
### AVL Tree
2023-11-16 13:43:45 +00:00
[Try it out ](https://vivid-algorithm.vercel.app/ ), or you can execute your own code using our [visual tool ](https://github.com/zrwusa/vivid-algorithm )
2023-09-18 14:04:39 +00:00
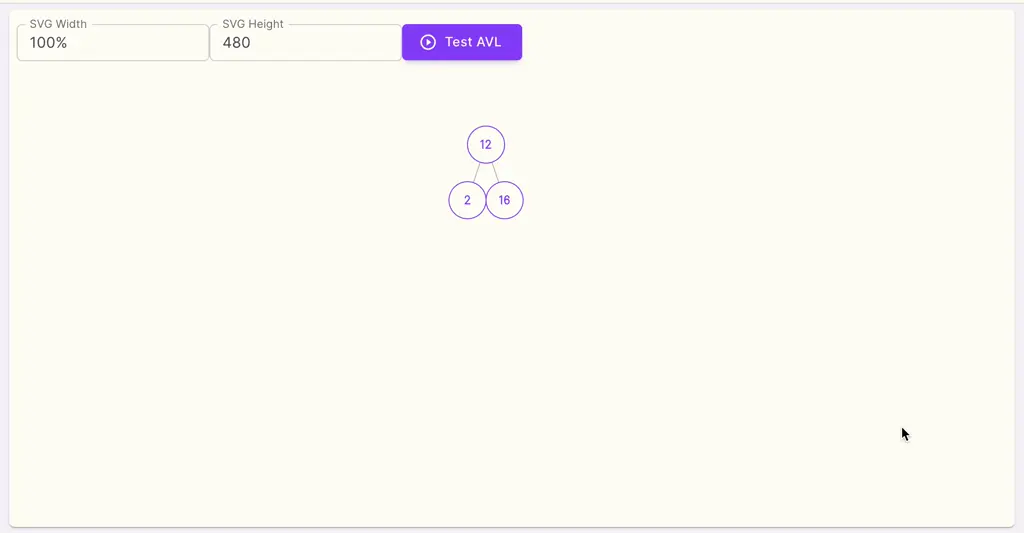
2023-11-16 13:43:45 +00:00
2023-11-17 02:47:13 +00:00
### Tree Multi Map
2023-11-16 13:43:45 +00:00
[Try it out ](https://vivid-algorithm.vercel.app/ ), or you can execute your own code using our [visual tool ](https://github.com/zrwusa/vivid-algorithm )
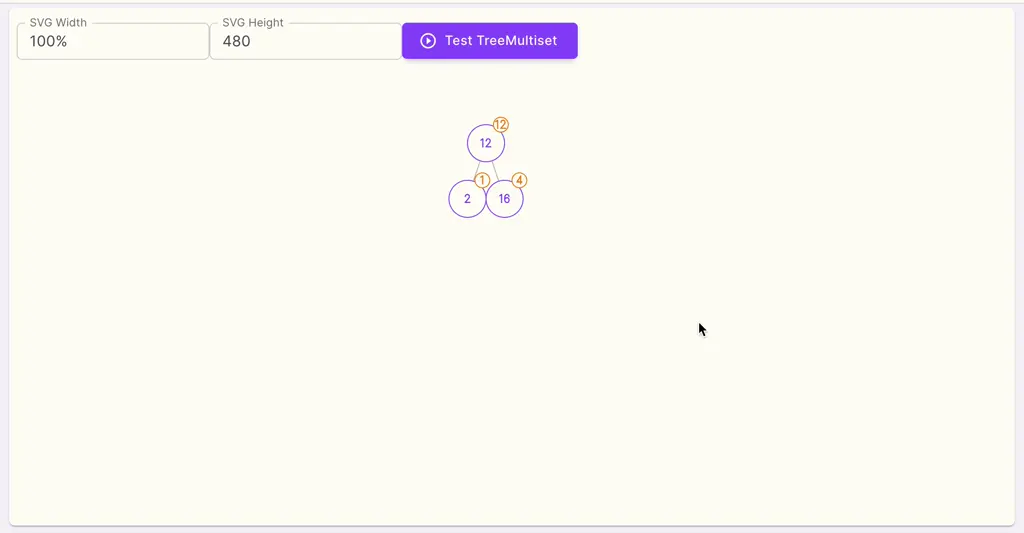
2023-11-17 02:47:13 +00:00
### Matrix
2023-11-16 13:43:45 +00:00
[Try it out ](https://vivid-algorithm.vercel.app/algorithm/graph/ ), or you can execute your own code using our [visual tool ](https://github.com/zrwusa/vivid-algorithm )
2023-09-18 14:04:39 +00:00
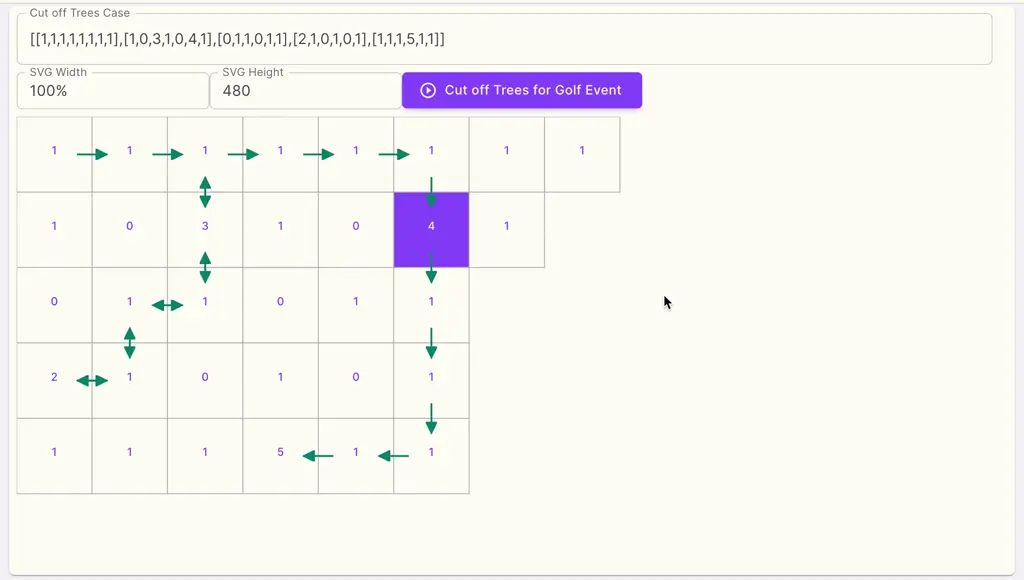
2023-11-16 13:43:45 +00:00
2023-11-17 02:47:13 +00:00
### Directed Graph
2023-11-16 13:43:45 +00:00
[Try it out ](https://vivid-algorithm.vercel.app/algorithm/graph/ ), or you can execute your own code using our [visual tool ](https://github.com/zrwusa/vivid-algorithm )
2023-09-18 14:04:39 +00:00
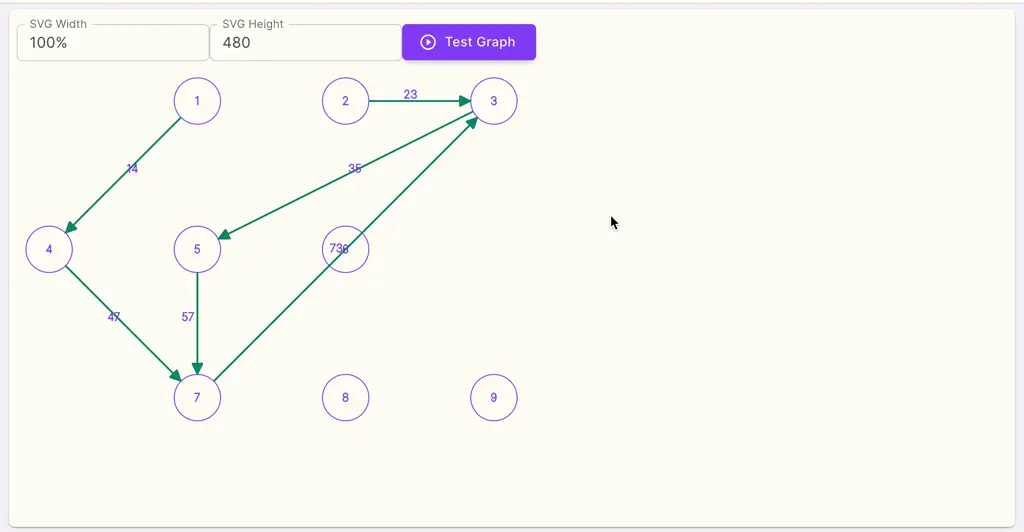
2023-11-16 13:43:45 +00:00
2023-11-17 02:47:13 +00:00
### Map Graph
2023-11-16 13:43:45 +00:00
[Try it out ](https://vivid-algorithm.vercel.app/algorithm/graph/ ), or you can execute your own code using our [visual tool ](https://github.com/zrwusa/vivid-algorithm )
2023-09-18 14:04:39 +00:00
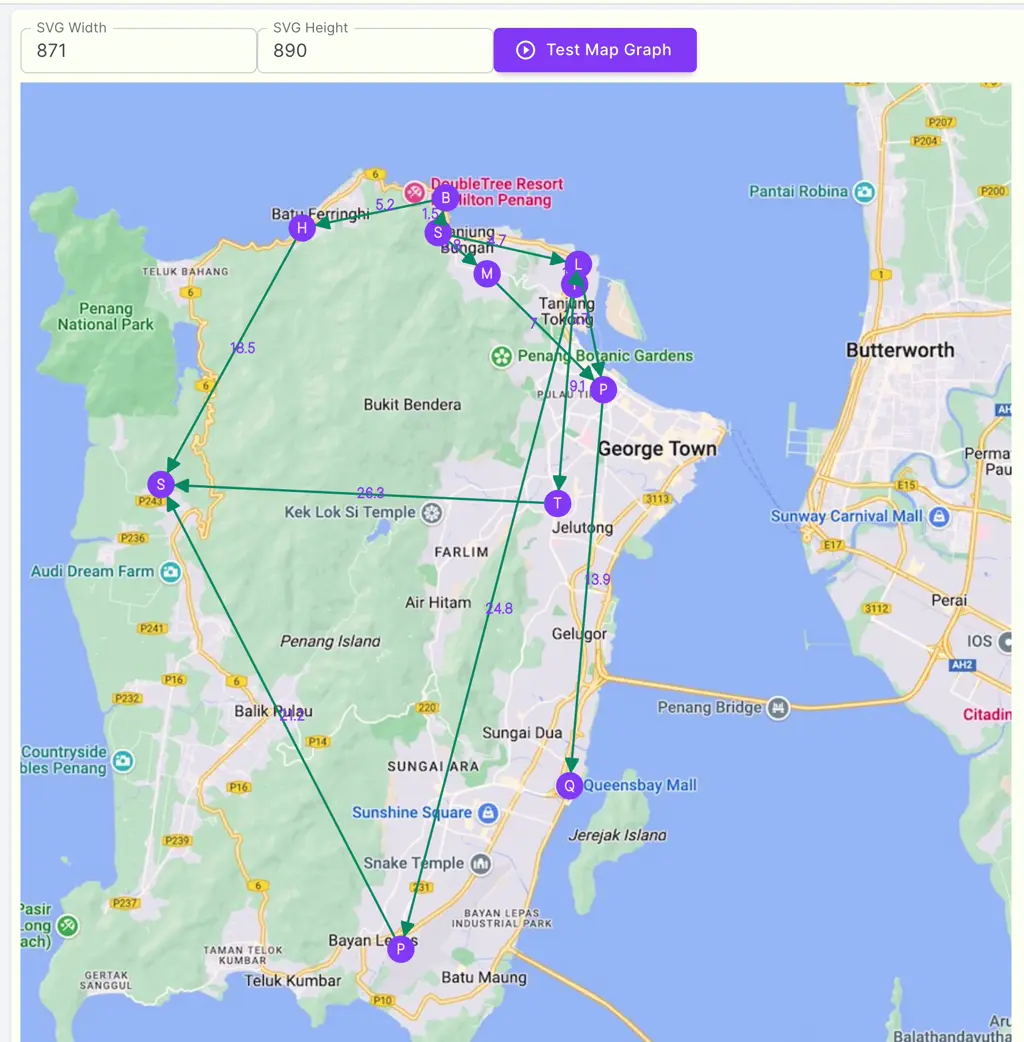
2023-09-09 15:49:53 +00:00
2023-08-28 12:04:43 +00:00
2023-11-16 13:43:45 +00:00
2023-11-08 12:25:02 +00:00
## Code Snippets
2023-09-24 13:31:09 +00:00
2023-08-28 12:04:43 +00:00
### Binary Search Tree (BST) snippet
2023-09-24 13:31:09 +00:00
2023-08-28 12:04:43 +00:00
#### TS
2023-09-24 13:31:09 +00:00
2023-09-22 03:20:33 +00:00
```ts
2023-09-24 13:31:09 +00:00
import {BST, BSTNode} from 'data-structure-typed';
const bst = new BST();
bst.add(11);
bst.add(3);
bst.addMany([15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5]);
bst.size === 16; // true
bst.has(6); // true
2023-11-08 12:25:02 +00:00
const node6 = bst.getNode(6); // BSTNode
2023-09-24 13:31:09 +00:00
bst.getHeight(6) === 2; // true
bst.getHeight() === 5; // true
bst.getDepth(6) === 3; // true
2023-11-08 12:25:02 +00:00
bst.getLeftMost()?.key === 1; // true
2023-09-24 13:31:09 +00:00
2023-10-28 07:58:48 +00:00
bst.delete(6);
2023-11-08 12:25:02 +00:00
bst.get(6); // undefined
2023-09-24 13:31:09 +00:00
bst.isAVLBalanced(); // true
2023-10-10 14:15:01 +00:00
bst.bfs()[0] === 11; // true
2023-09-24 13:31:09 +00:00
2023-11-08 12:25:02 +00:00
const objBST = new BST< {height: number, age: number}>();
objBST.add(11, { "name": "Pablo", "age": 15 });
objBST.add(3, { "name": "Kirk", "age": 1 });
objBST.addMany([15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5], [
{ "name": "Alice", "age": 15 },
{ "name": "Bob", "age": 1 },
{ "name": "Charlie", "age": 8 },
{ "name": "David", "age": 13 },
{ "name": "Emma", "age": 16 },
{ "name": "Frank", "age": 2 },
{ "name": "Grace", "age": 6 },
{ "name": "Hannah", "age": 9 },
{ "name": "Isaac", "age": 12 },
{ "name": "Jack", "age": 14 },
{ "name": "Katie", "age": 4 },
{ "name": "Liam", "age": 7 },
{ "name": "Mia", "age": 10 },
{ "name": "Noah", "age": 5 }
]
);
2023-09-24 13:31:09 +00:00
2023-10-28 07:58:48 +00:00
objBST.delete(11);
2023-08-28 12:04:43 +00:00
```
2023-09-24 13:31:09 +00:00
2023-08-28 12:04:43 +00:00
#### JS
2023-09-24 13:31:09 +00:00
2023-09-22 03:20:33 +00:00
```js
2023-09-24 13:31:09 +00:00
const {BST, BSTNode} = require('data-structure-typed');
const bst = new BST();
bst.add(11);
bst.add(3);
bst.addMany([15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5]);
2023-11-08 12:25:02 +00:00
bst.size === 16; // true
bst.has(6); // true
const node6 = bst.getNode(6);
bst.getHeight(6) === 2; // true
bst.getHeight() === 5; // true
bst.getDepth(6) === 3; // true
2023-09-24 13:31:09 +00:00
const leftMost = bst.getLeftMost();
2023-11-08 12:25:02 +00:00
leftMost?.key === 1; // true
2023-10-28 07:58:48 +00:00
bst.delete(6);
2023-11-08 12:25:02 +00:00
bst.get(6); // undefined
bst.isAVLBalanced(); // true or false
2023-10-10 14:15:01 +00:00
const bfsIDs = bst.bfs();
2023-11-08 12:25:02 +00:00
bfsIDs[0] === 11; // true
2023-09-24 13:31:09 +00:00
2023-08-28 12:04:43 +00:00
```
2023-09-20 03:39:24 +00:00
### AVLTree snippet
2023-09-24 13:31:09 +00:00
2023-09-20 03:39:24 +00:00
#### TS
2023-09-24 13:31:09 +00:00
2023-09-22 03:20:33 +00:00
```ts
import {AVLTree} from 'data-structure-typed';
const avlTree = new AVLTree();
avlTree.addMany([11, 3, 15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5])
avlTree.isAVLBalanced(); // true
2023-10-28 07:58:48 +00:00
avlTree.delete(10);
2023-09-22 03:20:33 +00:00
avlTree.isAVLBalanced(); // true
2023-09-20 03:39:24 +00:00
```
2023-09-24 13:31:09 +00:00
2023-09-20 03:39:24 +00:00
#### JS
2023-09-24 13:31:09 +00:00
2023-09-22 03:20:33 +00:00
```js
const {AVLTree} = require('data-structure-typed');
const avlTree = new AVLTree();
avlTree.addMany([11, 3, 15, 1, 8, 13, 16, 2, 6, 9, 12, 14, 4, 7, 10, 5])
avlTree.isAVLBalanced(); // true
2023-10-28 07:58:48 +00:00
avlTree.delete(10);
2023-09-22 03:20:33 +00:00
avlTree.isAVLBalanced(); // true
2023-09-20 03:39:24 +00:00
```
2023-08-28 12:04:43 +00:00
### Directed Graph simple snippet
2023-09-24 13:31:09 +00:00
2023-08-28 12:04:43 +00:00
#### TS or JS
2023-09-24 13:31:09 +00:00
2023-09-22 03:20:33 +00:00
```ts
2023-08-28 12:04:43 +00:00
import {DirectedGraph} from 'data-structure-typed';
2023-09-22 03:20:33 +00:00
const graph = new DirectedGraph();
graph.addVertex('A');
graph.addVertex('B');
graph.hasVertex('A'); // true
graph.hasVertex('B'); // true
graph.hasVertex('C'); // false
graph.addEdge('A', 'B');
graph.hasEdge('A', 'B'); // true
graph.hasEdge('B', 'A'); // false
2023-10-28 07:58:48 +00:00
graph.deleteEdgeSrcToDest('A', 'B');
2023-09-22 03:20:33 +00:00
graph.hasEdge('A', 'B'); // false
graph.addVertex('C');
graph.addEdge('A', 'B');
graph.addEdge('B', 'C');
2023-11-08 12:25:02 +00:00
const topologicalOrderKeys = graph.topologicalSort(); // ['A', 'B', 'C']
2023-08-28 12:04:43 +00:00
```
### Undirected Graph snippet
2023-09-24 13:31:09 +00:00
2023-08-28 12:04:43 +00:00
#### TS or JS
2023-09-24 13:31:09 +00:00
2023-09-22 03:20:33 +00:00
```ts
2023-08-28 12:04:43 +00:00
import {UndirectedGraph} from 'data-structure-typed';
2023-09-22 03:20:33 +00:00
const graph = new UndirectedGraph();
graph.addVertex('A');
graph.addVertex('B');
graph.addVertex('C');
graph.addVertex('D');
2023-10-28 07:58:48 +00:00
graph.deleteVertex('C');
2023-09-22 03:20:33 +00:00
graph.addEdge('A', 'B');
graph.addEdge('B', 'D');
const dijkstraResult = graph.dijkstra('A');
2023-11-08 12:25:02 +00:00
Array.from(dijkstraResult?.seen ?? []).map(vertex => vertex.key) // ['A', 'B', 'D']
2023-08-28 12:04:43 +00:00
```
2023-09-18 14:04:39 +00:00
2023-11-09 09:47:02 +00:00
## Built-in classic algorithms
< table >
< thead >
< tr >
< th > Algorithm< / th >
< th > Function Description< / th >
< th > Iteration Type< / th >
< / tr >
< / thead >
< tbody >
< tr >
< td > Binary Tree DFS< / td >
< td > Traverse a binary tree in a depth-first manner, starting from the root node, first visiting the left subtree,
and then the right subtree, using recursion.
< / td >
< td > Recursion + Iteration< / td >
< / tr >
< tr >
< td > Binary Tree BFS< / td >
< td > Traverse a binary tree in a breadth-first manner, starting from the root node, visiting nodes level by level
from left to right.
< / td >
< td > Iteration< / td >
< / tr >
< tr >
< td > Graph DFS< / td >
< td > Traverse a graph in a depth-first manner, starting from a given node, exploring along one path as deeply as
possible, and backtracking to explore other paths. Used for finding connected components, paths, etc.
< / td >
< td > Recursion + Iteration< / td >
< / tr >
< tr >
< td > Binary Tree Morris< / td >
< td > Morris traversal is an in-order traversal algorithm for binary trees with O(1) space complexity. It allows tree
traversal without additional stack or recursion.
< / td >
< td > Iteration< / td >
< / tr >
< tr >
< td > Graph BFS< / td >
< td > Traverse a graph in a breadth-first manner, starting from a given node, first visiting nodes directly connected
to the starting node, and then expanding level by level. Used for finding shortest paths, etc.
< / td >
< td > Recursion + Iteration< / td >
< / tr >
< tr >
< td > Graph Tarjan's Algorithm< / td >
< td > Find strongly connected components in a graph, typically implemented using depth-first search.< / td >
< td > Recursion< / td >
< / tr >
< tr >
< td > Graph Bellman-Ford Algorithm< / td >
< td > Finding the shortest paths from a single source, can handle negative weight edges< / td >
< td > Iteration< / td >
< / tr >
< tr >
< td > Graph Dijkstra's Algorithm< / td >
< td > Finding the shortest paths from a single source, cannot handle negative weight edges< / td >
< td > Iteration< / td >
< / tr >
< tr >
< td > Graph Floyd-Warshall Algorithm< / td >
< td > Finding the shortest paths between all pairs of nodes< / td >
< td > Iteration< / td >
< / tr >
< tr >
< td > Graph getCycles< / td >
< td > Find all cycles in a graph or detect the presence of cycles.< / td >
< td > Recursion< / td >
< / tr >
< tr >
< td > Graph getCutVertexes< / td >
< td > Find cut vertices in a graph, which are nodes that, when removed, increase the number of connected components in
the graph.
< / td >
< td > Recursion< / td >
< / tr >
< tr >
< td > Graph getSCCs< / td >
< td > Find strongly connected components in a graph, which are subgraphs where any two nodes can reach each other.
< / td >
< td > Recursion< / td >
< / tr >
< tr >
< td > Graph getBridges< / td >
< td > Find bridges in a graph, which are edges that, when removed, increase the number of connected components in the
graph.
< / td >
< td > Recursion< / td >
< / tr >
< tr >
< td > Graph topologicalSort< / td >
< td > Perform topological sorting on a directed acyclic graph (DAG) to find a linear order of nodes such that all
directed edges go from earlier nodes to later nodes.
< / td >
< td > Recursion< / td >
< / tr >
< / tbody >
< / table >
## API docs & Examples
[API Docs ](https://data-structure-typed-docs.vercel.app )
[Live Examples ](https://vivid-algorithm.vercel.app )
< a href = "https://github.com/zrwusa/vivid-algorithm" target = "_blank" > Examples Repository< / a >
2023-08-16 16:50:14 +00:00
## Data Structures
2023-09-24 13:31:09 +00:00
2023-08-22 14:50:16 +00:00
< table >
< thead >
< tr >
< th > Data Structure< / th >
< th > Unit Test< / th >
< th > Performance Test< / th >
< th > API Documentation< / th >
< th > Implemented< / th >
< / tr >
< / thead >
< tbody >
< tr >
< td > Binary Tree< / td >
2023-09-09 15:49:53 +00:00
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-08-22 14:50:16 +00:00
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/BinaryTree.html" > < span > Binary Tree< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Binary Search Tree (BST)< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/BST.html" > < span > BST< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > AVL Tree< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/AVLTree.html" > < span > AVLTree< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
2023-11-06 03:01:32 +00:00
< td > Red Black Tree< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-11-09 09:47:02 +00:00
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/RedBlackTree.html" > < span > RedBlackTree< / span > < / a > < / td >
2023-11-06 03:01:32 +00:00
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
2023-08-22 14:50:16 +00:00
< td > Tree Multiset< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-11-08 01:26:43 +00:00
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/TreeMultimap.html" > < span > TreeMultimap< / span > < / a > < / td >
2023-08-22 14:50:16 +00:00
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Segment Tree< / td >
2023-11-06 03:01:32 +00:00
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-08-22 14:50:16 +00:00
< td > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/SegmentTree.html" > < span > SegmentTree< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Binary Indexed Tree< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/BinaryIndexedTree.html" > < span > BinaryIndexedTree< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Graph< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/AbstractGraph.html" > < span > AbstractGraph< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Directed Graph< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/DirectedGraph.html" > < span > DirectedGraph< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Undirected Graph< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/UndirectedGraph.html" > < span > UndirectedGraph< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Linked List< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/SinglyLinkedList.html" > < span > SinglyLinkedList< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Singly Linked List< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/SinglyLinkedList.html" > < span > SinglyLinkedList< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Doubly Linked List< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/DoublyLinkedList.html" > < span > DoublyLinkedList< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Queue< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/Queue.html" > < span > Queue< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Object Deque< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/ObjectDeque.html" > < span > ObjectDeque< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Array Deque< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/ArrayDeque.html" > < span > ArrayDeque< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Stack< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-11-06 03:01:32 +00:00
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-08-22 14:50:16 +00:00
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/Stack.html" > < span > Stack< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Coordinate Set< / td >
2023-11-06 03:01:32 +00:00
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-08-22 14:50:16 +00:00
< td > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/CoordinateSet.html" > < span > CoordinateSet< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Coordinate Map< / td >
2023-11-06 03:01:32 +00:00
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-08-22 14:50:16 +00:00
< td > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/CoordinateMap.html" > < span > CoordinateMap< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Heap< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/Heap.html" > < span > Heap< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Priority Queue< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/PriorityQueue.html" > < span > PriorityQueue< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Max Priority Queue< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/MaxPriorityQueue.html" > < span > MaxPriorityQueue< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Min Priority Queue< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/MinPriorityQueue.html" > < span > MinPriorityQueue< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< tr >
< td > Trie< / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-11-06 03:01:32 +00:00
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
2023-08-22 14:50:16 +00:00
< td > < a href = "https://data-structure-typed-docs.vercel.app/classes/Trie.html" > < span > Trie< / span > < / a > < / td >
< td > < img src = "https://raw.githubusercontent.com/zrwusa/assets/master/images/data-structure-typed/assets/tick.svg" alt = "" > < / td >
< / tr >
< / tbody >
< / table >
2023-09-26 09:09:28 +00:00
2023-11-17 02:47:13 +00:00
## Standard library data structure comparison
2023-09-26 09:09:28 +00:00
2023-10-10 09:22:18 +00:00
2023-09-26 09:09:28 +00:00
< table >
< thead >
2023-10-10 09:22:18 +00:00
< tr >
< th > Data Structure Typed< / th >
2023-11-03 17:21:30 +00:00
< th > C++ STL< / th >
2023-10-10 09:22:18 +00:00
< th > java.util< / th >
< th > Python collections< / th >
< / tr >
2023-09-26 09:09:28 +00:00
< / thead >
< tbody >
2023-10-10 09:22:18 +00:00
< tr >
< td > DoublyLinkedList< E> < / td >
< td > list< T> < / td >
< td > LinkedList< E> < / td >
< td > deque< / td >
< / tr >
< tr >
< td > SinglyLinkedList< E> < / td >
< td > -< / td >
< td > -< / td >
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > Array< E> < / td >
< td > vector< T> < / td >
< td > ArrayList< E> < / td >
< td > list< / td >
2023-10-10 09:22:18 +00:00
< / tr >
< tr >
< td > Queue< E> < / td >
< td > queue< T> < / td >
< td > Queue< E> < / td >
< td > -< / td >
< / tr >
2023-11-08 12:25:02 +00:00
< tr >
< td > Deque< E> < / td >
< td > deque< T> < / td >
< td > -< / td >
< td > -< / td >
< / tr >
2023-10-10 09:22:18 +00:00
< tr >
< td > PriorityQueue< E> < / td >
< td > priority_queue< T> < / td >
< td > PriorityQueue< E> < / td >
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > Heap< E> < / td >
2023-10-10 09:22:18 +00:00
< td > priority_queue< T> < / td >
< td > PriorityQueue< E> < / td >
< td > heapq< / td >
< / tr >
< tr >
< td > Stack< E> < / td >
< td > stack< T> < / td >
< td > Stack< E> < / td >
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > Set< E> < / td >
< td > set< T> < / td >
< td > HashSet< E> < / td >
< td > set< / td >
< / tr >
< tr >
< td > Map< K, V> < / td >
< td > map< K, V> < / td >
< td > HashMap< K, V> < / td >
< td > dict< / td >
2023-10-10 09:22:18 +00:00
< / tr >
< tr >
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > unordered_set< T> < / td >
< td > HashSet< E> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< / tr >
< tr >
< td > HashMap< K, V> < / td >
< td > unordered_map< K, V> < / td >
< td > HashMap< K, V> < / td >
< td > defaultdict< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > Map< K, V> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > OrderedDict< / td >
2023-10-10 09:22:18 +00:00
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > BinaryTree< K, V> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< td > -< / td >
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > BST< K, V> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< td > -< / td >
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > TreeMultimap< K, V> < / td >
< td > multimap< K, V> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > AVLTree< E> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > TreeSet< E> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > AVLTree< K, V> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > TreeMap< K, V> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
2023-11-08 12:25:02 +00:00
< / tr >
< tr >
< td > AVLTree< E> < / td >
< td > set< / td >
< td > TreeSet< E> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > Trie< / td >
< td > -< / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< td > -< / td >
< / tr >
< tr >
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > multiset< T> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > DirectedGraph< V, E> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< td > -< / td >
< td > -< / td >
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > UndirectedGraph< V, E> < / td >
< td > -< / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< td > -< / td >
< / tr >
< tr >
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > unordered_multiset< / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > Counter< / td >
2023-10-10 09:22:18 +00:00
< / tr >
< tr >
2023-11-08 12:25:02 +00:00
< td > -< / td >
< td > -< / td >
< td > LinkedHashSet< E> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< / tr >
< tr >
< td > -< / td >
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > LinkedHashMap< K, V> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< / tr >
< tr >
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > unordered_multimap< K, V> < / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< td > -< / td >
< / tr >
< tr >
< td > -< / td >
2023-11-08 12:25:02 +00:00
< td > bitset< N> < / td >
< td > -< / td >
2023-10-10 09:22:18 +00:00
< td > -< / td >
< / tr >
2023-09-26 09:09:28 +00:00
< / tbody >
< / table >
2023-11-17 02:47:13 +00:00
## Benchmark
[//]: # (No deletion!!! Start of Replace Section)
< div class = "json-to-html-collapse clearfix 0" >
2023-11-17 08:11:31 +00:00
< div class = 'collapsible level0' > < span class = 'json-to-html-label' > deque< / span > < / div >
2023-11-17 10:44:14 +00:00
< div class = "content" > < table style = "display: table; width:100%; table-layout: fixed;" > < tr > < th > test name< / th > < th > time taken (ms)< / th > < th > executions per sec< / th > < th > sample deviation< / th > < / tr > < tr > < td > 1,000,000 push< / td > < td > 70.01< / td > < td > 14.28< / td > < td > 0.01< / td > < / tr > < tr > < td > 1,000,000 CPT push< / td > < td > 14.52< / td > < td > 68.86< / td > < td > 0.00< / td > < / tr > < tr > < td > 1,000,000 push & pop< / td > < td > 72.74< / td > < td > 13.75< / td > < td > 0.01< / td > < / tr > < tr > < td > 1,000,000 push & shift< / td > < td > 74.59< / td > < td > 13.41< / td > < td > 0.01< / td > < / tr > < tr > < td > 1,000,000 unshift & shift< / td > < td > 72.73< / td > < td > 13.75< / td > < td > 0.01< / td > < / tr > < / table > < / div >
2023-11-17 02:47:13 +00:00
< / div >
[//]: # (No deletion!!! End of Replace Section)
2023-09-24 13:31:09 +00:00
2023-11-17 02:47:13 +00:00
## Codebase design
### Adhere to ES6 and ESNext standard naming conventions for APIs.
2023-10-21 12:03:54 +00:00
Standardize API conventions by using 'add' and 'delete' for element manipulation methods in all data structures.
Opt for concise and clear method names, avoiding excessive length while ensuring explicit intent.
### Object-oriented programming(OOP)
2023-11-08 01:26:43 +00:00
By strictly adhering to object-oriented design (BinaryTree -> BST -> AVLTree -> TreeMultimap), you can seamlessly
2023-09-24 13:31:09 +00:00
inherit the existing data structures to implement the customized ones you need. Object-oriented design stands as the
optimal approach to data structure design.